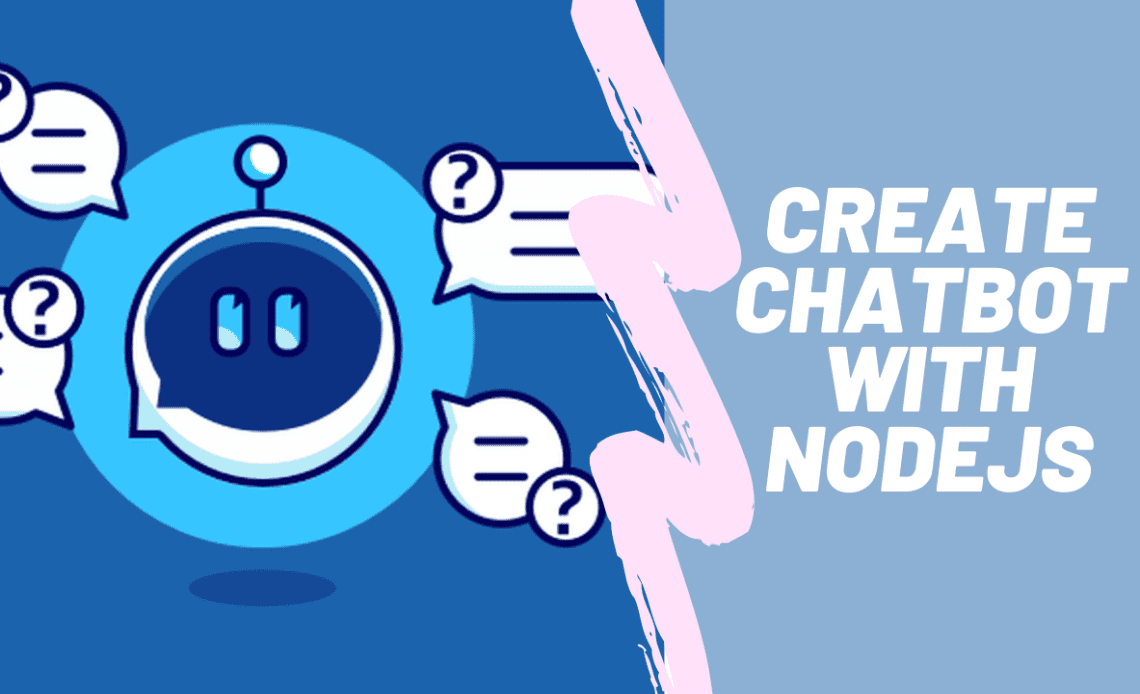
Sure, I can help you build a chatbot with Node.js! Here are the steps you can follow:
- Set up a Node.js project: First, create a new directory for your project and navigate into it using the command line. Then, run
npm init
to create a newpackage.json
file for your project. - Install dependencies: Next, install the necessary dependencies for your chatbot. You can use a package manager like
npm
to install the required packages. For example, you may want to use thebotbuilder
package to build your chatbot:
npm install botbuilder
- Set up the chatbot: Once you have the necessary dependencies installed, you can start building your chatbot. You’ll need to define the behavior of your chatbot using the Bot Builder SDK. Here’s an example of how you can create a simple echo bot that repeats back whatever the user says:
const { ActivityHandler, MessageFactory } = require('botbuilder');
class EchoBot extends ActivityHandler {
async onMessage(context) {
const message = MessageFactory.text(`You said "${context.activity.text}"`);
await context.sendActivity(message);
}
}
module.exports.EchoBot = EchoBot;
In this example, we define a new class called EchoBot
that extends ActivityHandler
from the botbuilder
package. The onMessage
method is called whenever the user sends a message to the bot. It simply creates a new message that repeats back the user’s input and sends it back to the user.
- Run the chatbot: Finally, you can start your chatbot by running the following code:
const { EchoBot } = require('./echoBot');
const adapter = new BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
const bot = new EchoBot();
adapter.onTurnError = async (context, error) => {
console.error(`Error processing turn: ${ error }`);
await context.sendActivity(`Oops! Something went wrong.`);
};
const server = restify.createServer();
server.use(restify.plugins.bodyParser());
server.post('/api/messages', (req, res) => {
adapter.processActivity(req, res, async (context) => {
await bot.run(context);
});
});
server.listen(process.env.port || process.env.PORT || 3978, () => {
console.log(`\n${ server.name } listening to ${ server.url }`);
});
This code sets up an HTTP server using the restify
package, and listens for incoming requests to the /api/messages
endpoint. When a request is received, it passes it to the BotFrameworkAdapter
from the botbuilder
package, which processes the incoming activity and passes it to the EchoBot
instance.
That’s it! You now have a simple chatbot that can echo back whatever the user says. Of course, you’ll likely want to customize the behavior of your chatbot and add more features. But this should give you a good starting point.