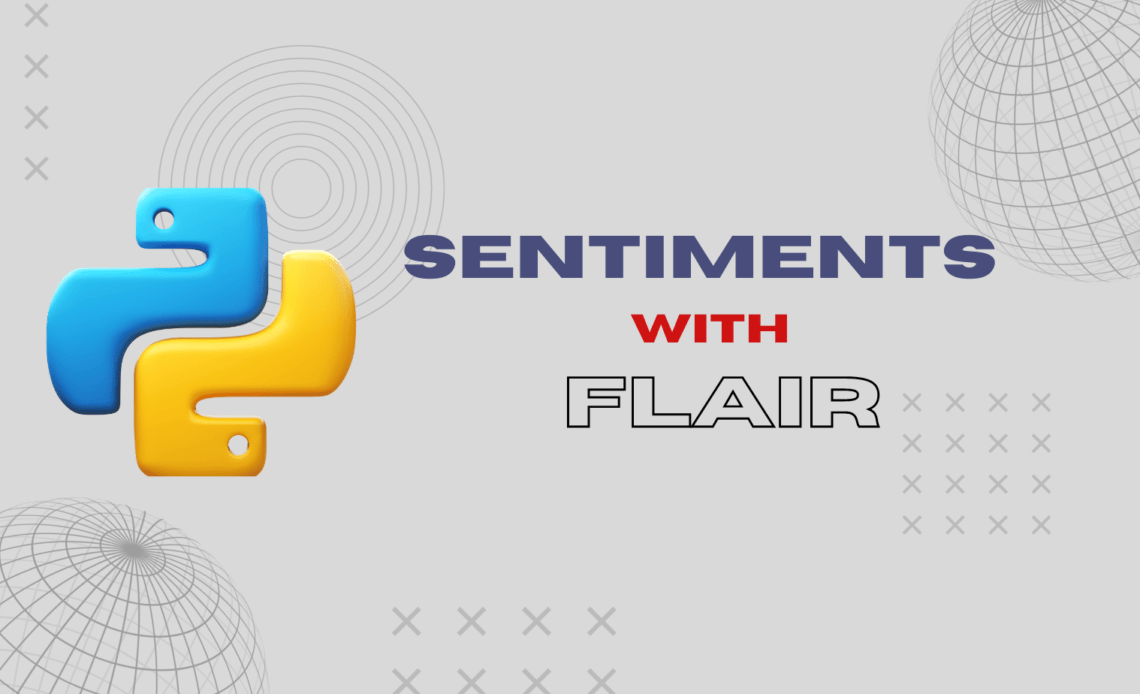
Sentiment analysis is a powerful technique in natural language processing (NLP) that involves determining the emotional tone behind a piece of text. Whether you’re monitoring social media, analyzing customer reviews, or gauging public opinion, understanding sentiment can provide valuable insights. In this blog post, we’ll explore how to perform sentiment analysis using the Flair package in Python.
What is Flair?
Flair is an open-source NLP library that makes it easy to apply state-of-the-art natural language processing models to your text data. It is built on top of popular deep learning frameworks such as PyTorch. Flair not only supports various NLP tasks but also comes with pre-trained models that are ready to use out of the box.
Installing Flair
Before we dive into sentiment analysis, let’s install the Flair package:
pip install flair
Getting Started with Flair Sentiment Analysis
Flair simplifies sentiment analysis with its easy-to-use interface. Let’s walk through a simple example:
from flair.models import TextClassifier
from flair.data import Sentence
# Load the pre-trained sentiment classifier
classifier = TextClassifier.load('en-sentiment')
# Create a Sentence object
sentence = Sentence('Flair makes natural language processing easy and fun!')
# Predict the sentiment
classifier.predict(sentence)
# Print the sentiment value (POSITIVE, NEGATIVE, or NEUTRAL) and confidence score
print(f"Sentiment: {sentence.labels[0].value} (Confidence: {sentence.labels[0].score})")
In this example, we loaded the pre-trained English sentiment classifier and applied it to a sample sentence. The predict
method assigns a sentiment label to the sentence, and we can access the result through the labels
attribute.
Customizing Sentiment Analysis
Flair allows you to train custom sentiment classifiers on your own data. If the pre-trained models don’t fit your specific domain, you can fine-tune or train new models to achieve better accuracy.
Fine-tuning the Model
To fine-tune a sentiment model on your data, you need labeled examples. Flair provides tools to create your own dataset and train a model:
from flair.datasets import CSVClassificationCorpus
from flair.trainers import ModelTrainer
# Define your CSV file with labeled data
corpus = CSVClassificationCorpus('your_data.csv', {'text_column': 0, 'label_column': 1})
# Load pre-trained sentiment classifier
classifier = TextClassifier.load('en-sentiment')
# Create a trainer for fine-tuning
trainer = ModelTrainer(classifier, corpus)
# Fine-tune the model
trainer.train('path/to/save/fine-tuned-model', max_epochs=5)
Training a Model from Scratch
If you have a large labeled dataset, you can train a sentiment model from scratch:
from flair.models import TextClassifier
from flair.trainers import ModelTrainer
from flair.datasets import ClassificationCorpus
# Define your custom dataset
corpus = ClassificationCorpus(
path_to_train_data='path/to/train',
path_to_test_data='path/to/test',
path_to_dev_data='path/to/dev'
)
# Create a TextClassifier with your own parameters
classifier = TextClassifier(document_embeddings, label_dictionary, multi_label=False)
# Create a trainer for training from scratch
trainer = ModelTrainer(classifier, corpus)
# Train the model
trainer.train('path/to/save/trained-model', max_epochs=10)
Conclusion
Flair simplifies sentiment analysis in Python, providing both pre-trained models for quick implementation and the flexibility to fine-tune or train models from scratch. Whether you’re a beginner or an NLP enthusiast, Flair’s intuitive API and extensive documentation make it a valuable tool in your sentiment analysis toolkit.
Start exploring Flair today and unlock the power of sentiment analysis in your projects!