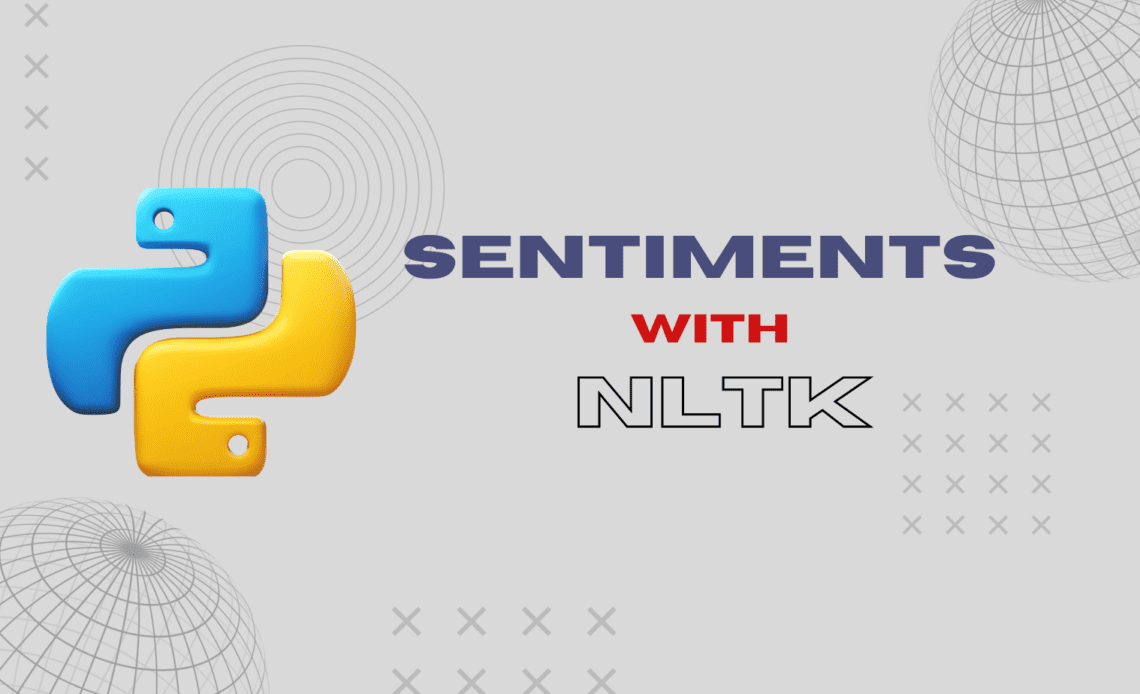
Sentiment analysis, also known as opinion mining, is a powerful application of natural language processing that involves determining the sentiment expressed in a piece of text. Whether it’s understanding customer reviews, social media comments, or news articles, sentiment analysis can provide valuable insights into the emotions and opinions conveyed in textual data.
Getting Started with NLTK
The NLTK library in Python is a treasure trove of tools and resources for natural language processing tasks. To perform sentiment analysis, we can leverage NLTK’s built-in functionalities, including pre-trained models and datasets.
Installing NLTK
If you haven’t installed NLTK yet, you can do so using the following command:
pip install nltk
Example: Analyzing Sentiment of Movie Reviews
Let’s dive into a practical example by analyzing the sentiment of movie reviews. NLTK provides a dataset of movie reviews labeled with positive and negative sentiments.
import nltk
from nltk.corpus import movie_reviews
from nltk import FreqDist
from nltk import classify
from nltk import NaiveBayesClassifier
# Download the movie_reviews dataset
nltk.download('movie_reviews')
# Prepare the data
documents = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
# Shuffle the documents
import random
random.shuffle(documents)
# Extract features from the words
all_words = FreqDist(w.lower() for w in movie_reviews.words())
word_features = list(all_words.keys())[:3000]
def document_features(document):
document_words = set(document)
features = {word: (word in document_words) for word in word_features}
return features
featuresets = [(document_features(d), c) for (d, c) in documents]
train_set, test_set = featuresets[:1900], featuresets[1900:]
# Train a Naive Bayes classifier
classifier = NaiveBayesClassifier.train(train_set)
# Test the classifier
accuracy = classify.accuracy(classifier, test_set)
print(f'Classifier Accuracy: {accuracy}')
# Test the classifier with a custom review
custom_review = "The movie was fantastic! The plot was gripping, and the characters were well-developed."
custom_features = document_features(custom_review.split())
sentiment = classifier.classify(custom_features)
print(f"Predicted Sentiment: {sentiment}")
In this example, we use a Naive Bayes classifier to analyze the sentiment of movie reviews. The classifier is trained on a dataset of positive and negative reviews, and then we test it with a custom review.
Conclusion
Sentiment analysis is a valuable tool for understanding the emotions and opinions expressed in textual data. NLTK provides a robust framework for performing sentiment analysis in Python, making it accessible for both beginners and experienced NLP practitioners.
In this blog post, we explored the basics of sentiment analysis using NLTK and demonstrated how to train a simple classifier to analyze the sentiment of movie reviews. The ability to discern sentiment opens up a world of possibilities for extracting valuable insights from text data, ranging from customer feedback to social media sentiments.
Feel free to explore more advanced techniques and datasets to enhance your sentiment analysis capabilities using NLTK!