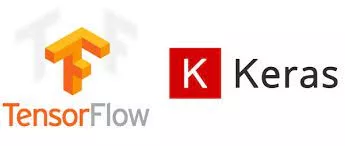
Are you ready to dive into the exciting world of deep learning? Look no further than Keras, a high-level neural networks API written in Python. In this comprehensive guide, we’ll walk you through the basics of Keras and show you how to kickstart your journey into the realm of artificial intelligence.
Understanding the Basics
What is Keras?
Keras is an open-source deep learning library that facilitates the creation and training of neural networks. One of its key advantages is its user-friendly interface, making it an ideal choice for beginners and experienced developers alike.
Installation Made Easy
Getting started with Keras is a breeze. You can install it using pip, the Python package installer:
pip install keras
Ensure you have a backend engine such as TensorFlow or Theano installed, as Keras relies on them for its computations.
Building Your First Neural Network
Let’s create a simple neural network to get a feel for how Keras works. Open your favorite Python environment and follow along:
# Importing necessary libraries
from keras.models import Sequential
from keras.layers import Dense
# Creating a sequential model
model = Sequential()
# Adding a densely connected layer with 64 units and input shape of (100,)
model.add(Dense(64, input_shape=(100,), activation='relu'))
# Adding an output layer with one unit (binary classification)
model.add(Dense(1, activation='sigmoid'))
# Compiling the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
Congratulations! You’ve just built a simple neural network using Keras.
Training Your Model
Next, let’s train our model using some example data:
# Assuming you have training data (X_train, y_train)
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_split=0.2)
This code snippet trains the model for 10 epochs with a batch size of 32, using 20% of the data for validation.
Evaluating Model Performance
Once trained, it’s time to evaluate the model:
# Assuming you have test data (X_test, y_test)
loss, accuracy = model.evaluate(X_test, y_test)
print(f"Test Loss: {loss}, Test Accuracy: {accuracy}")
Conclusion
In this guide, we’ve covered the basics of Keras, from installation to building and training your first neural network. As you continue your deep learning journey, explore the vast capabilities of Keras and its integration with powerful backend engines like TensorFlow.
Remember, practice is key to mastering any new skill. Experiment with different architectures, datasets, and hyperparameters to deepen your understanding of Keras and unleash its full potential.
Happy coding!