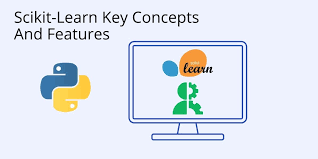
Introduction
In the rapidly evolving field of data science, mastering machine learning is crucial for unlocking valuable insights from data. One of the most powerful and user-friendly libraries for machine learning in Python is Scikit-Learn. In this comprehensive guide, we will explore the key features of Scikit-Learn and provide practical examples to help you leverage its capabilities effectively.
Chapter 1: Getting Started with Scikit-Learn
Understanding the Basics
Scikit-Learn is an open-source machine learning library that provides simple and efficient tools for data analysis and modeling. Its versatility makes it an ideal choice for both beginners and experienced data scientists.
To get started, make sure you have Scikit-Learn installed:
pip install scikit-learn
Chapter 2: Exploring Scikit-Learn’s Core Functionality
Data Preprocessing with Scikit-Learn
Before diving into machine learning models, it’s essential to preprocess your data. Scikit-Learn offers a range of tools for data cleaning, normalization, and transformation. Let’s look at an example of handling missing data:
from sklearn.impute import SimpleImputer
# Create a SimpleImputer object
imputer = SimpleImputer(strategy='mean')
# Fit and transform the data
X_imputed = imputer.fit_transform(X)
Chapter 3: Building Your First Machine Learning Model
Linear Regression with Scikit-Learn
Linear regression is a fundamental machine learning algorithm. Let’s build a simple linear regression model using Scikit-Learn:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a linear regression model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
Chapter 4: Fine-Tuning Your Model
Hyperparameter Tuning with Scikit-Learn
Optimizing your model’s performance involves fine-tuning its hyperparameters. Scikit-Learn provides tools like GridSearchCV
for this purpose. Let’s see an example with a support vector machine (SVM):
from sklearn.model_selection import GridSearchCV
from sklearn.svm import SVR
# Define the hyperparameters to tune
param_grid = {'C': [0.1, 1, 10], 'kernel': ['linear', 'rbf', 'poly']}
# Create an SVM model
svm_model = SVR()
# Use GridSearchCV for hyperparameter tuning
grid_search = GridSearchCV(svm_model, param_grid, cv=3)
grid_search.fit(X_train, y_train)
# Get the best hyperparameters
best_params = grid_search.best_params_
print(f'Best Hyperparameters: {best_params}')
Conclusion
Scikit-Learn is a powerful ally in your journey to becoming a proficient data scientist. By following this comprehensive guide, you’ve learned the basics, explored core functionality, built your first machine learning model, and fine-tuned it for optimal performance.
Remember, continuous learning and hands-on practice are key to mastering machine learning with Scikit-Learn. Stay curious, explore different algorithms, and apply your knowledge to real-world projects.
Start implementing what you’ve learned, and watch your data science skills soar to new heights with Scikit-Learn!