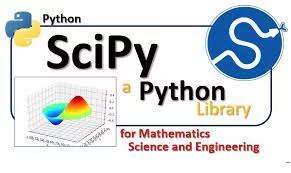
Introduction
In the realm of scientific and technical computing, Python stands out as a versatile and powerful language. One of its key assets is the SciPy library, a fundamental tool for scientific computing tasks. In this comprehensive guide, we will explore the features and capabilities of SciPy, providing practical examples to showcase its prowess.
What is SciPy?
SciPy is an open-source library that builds on NumPy, providing additional functionality for optimization, integration, interpolation, eigenvalue problems, and more. It is an essential component of the scientific Python ecosystem and is widely used in academia, research, and industry.
Installation
Before we dive into the examples, let’s ensure you have SciPy installed. You can install it using pip:
pip install scipy
Example 1: Solving Linear Equations
SciPy’s linalg
module is a powerhouse for linear algebra operations. Let’s solve a system of linear equations using SciPy:
import numpy as np
from scipy.linalg import solve
# Coefficient matrix
A = np.array([[2, 1], [1, -3]])
# Right-hand side
b = np.array([8, -3])
# Solve the system of linear equations
solution = solve(A, b)
print("Solution:", solution)
This example demonstrates SciPy’s ability to efficiently solve linear equations, a common task in various scientific disciplines.
Example 2: Curve Fitting with SciPy
Curve fitting is crucial in data analysis and modeling. SciPy’s curve_fit
function makes this task straightforward. Consider the following example:
import numpy as np
import matplotlib.pyplot as plt
from scipy.optimize import curve_fit
# Define the true function
def true_function(x, a, b):
return a * x + b
# Generate synthetic data
x_data = np.linspace(0, 10, 50)
y_data = true_function(x_data, 2, 1) + np.random.normal(0, 1, size=len(x_data))
# Fit the curve to the data
params, covariance = curve_fit(true_function, x_data, y_data)
# Plot the results
plt.scatter(x_data, y_data, label='Data')
plt.plot(x_data, true_function(x_data, *params), color='red', label='Fitted curve')
plt.legend()
plt.show()
This example illustrates how SciPy facilitates curve fitting, enabling researchers to extract meaningful insights from experimental or observational data.
Conclusion
SciPy plays a pivotal role in the Python ecosystem for scientific computing. This guide provided a glimpse into its capabilities, showcasing examples of linear equation solving and curve fitting. As you delve deeper into your scientific endeavors, consider SciPy as a reliable companion, streamlining complex computations with ease.
By integrating SciPy into your workflow, you unlock a world of possibilities for scientific and technical computing in Python. Embrace the power of SciPy and elevate your coding experience to new heights!
Remember to explore the official SciPy documentation for a comprehensive understanding of its features and functions.