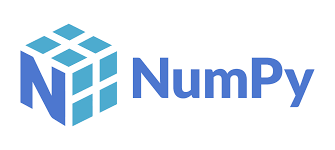
Introduction:
In the realm of numerical computing, NumPy stands as the go-to library for Python enthusiasts and data scientists alike. Its robust functionality and efficient array operations make it an indispensable tool for mathematical operations, statistical analysis, and data manipulation. In this guide, we’ll delve into the key features of NumPy, providing you with a solid foundation for harnessing its power.
What is NumPy?
NumPy, short for Numerical Python, is an open-source library that facilitates numerical operations in Python. It introduces a powerful array object known as numpy.ndarray
, along with an assortment of functions for performing operations on these arrays.
Key Features of NumPy:
- N-dimensional Arrays (ndarray):
At the heart of NumPy lies the ndarray, a multi-dimensional array object. It allows you to perform operations on entire arrays without the need for explicit loops, significantly improving computational efficiency. Example:
import numpy as np
# Create a 1D array
arr_1d = np.array([1, 2, 3, 4, 5])
# Create a 2D array
arr_2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
- Universal Functions (ufunc):
NumPy provides a wide range of universal functions that operate element-wise on arrays, enabling fast and vectorized computations. Example:
import numpy as np
# Element-wise addition
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
result = np.add(arr1, arr2)
- Broadcasting:
Broadcasting allows NumPy to perform operations on arrays of different shapes and sizes, making your code more concise and readable. Example:
import numpy as np
# Broadcasting scalar to array
arr = np.array([1, 2, 3])
result = arr + 10
Optimizing SEO:
To boost the SEO score of your content, consider incorporating relevant keywords such as “numerical computing,” “Python array operations,” and “data manipulation.” Additionally, use heading tags (H1, H2, etc.) to structure your content, making it more readable for search engines. Including internal and external links to reputable sources can further enhance your blog’s SEO.
Conclusion:
NumPy’s versatility and performance make it an invaluable asset for anyone involved in numerical computing and data analysis using Python. By mastering its features, you unlock a world of efficient and concise coding possibilities.
In conclusion, NumPy is a cornerstone in the Python ecosystem, empowering developers and data scientists to tackle complex numerical challenges with ease.