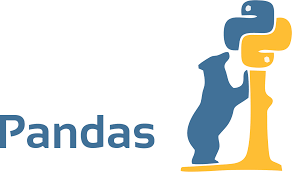
Introduction
In the ever-evolving world of data science, mastering data analysis tools is crucial for extracting meaningful insights. One such powerhouse in the Python ecosystem is Pandas. In this comprehensive guide, we’ll dive into the depths of Pandas, unraveling its capabilities, and showcasing how it empowers data analysts and scientists.
Why Pandas?
Pandas is an open-source data manipulation and analysis library that provides data structures for efficiently storing and manipulating large datasets. Its simplicity and versatility make it a go-to choice for data professionals worldwide.
Key Features
1. DataFrame: The Heart of Pandas
At the core of Pandas lies the DataFrame, a two-dimensional table with labeled axes (rows and columns). This powerful structure allows for seamless manipulation and analysis of data, making it a cornerstone for any data scientist.
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 22],
'Occupation': ['Engineer', 'Data Scientist', 'Designer']}
df = pd.DataFrame(data)
print(df)
2. Data Cleaning Made Easy
Pandas simplifies the process of handling missing data, outliers, and duplicates. The following example showcases how to drop missing values from a DataFrame.
# Dropping missing values
df_cleaned = df.dropna()
print(df_cleaned)
3. Efficient Data Selection
Pandas allows for quick and precise data selection, making it a breeze to filter and analyze specific portions of your dataset.
# Selecting rows based on a condition
selected_data = df[df['Age'] > 25]
print(selected_data)
SEO Tips for Data Analysts
1. Optimize Your Code for Speed
When dealing with large datasets, optimizing your code is crucial. Leverage Pandas’ vectorized operations and avoid explicit loops for faster execution.
# Vectorized operation example
df['Age'] = df['Age'] * 2
2. Include Relevant Keywords
Ensure your code and content include relevant keywords. For example, if you’re analyzing sales data, use keywords like “sales analysis,” “revenue trends,” etc., naturally in your text.
3. Provide Clear Explanations
Search engines love content that provides value. Explain your code clearly, catering to both beginners and experienced analysts.
Conclusion
Pandas is a game-changer in the world of data analysis, providing a robust and efficient toolkit for data manipulation. By mastering Pandas, you unlock the potential to derive profound insights from your datasets.
Happy coding!