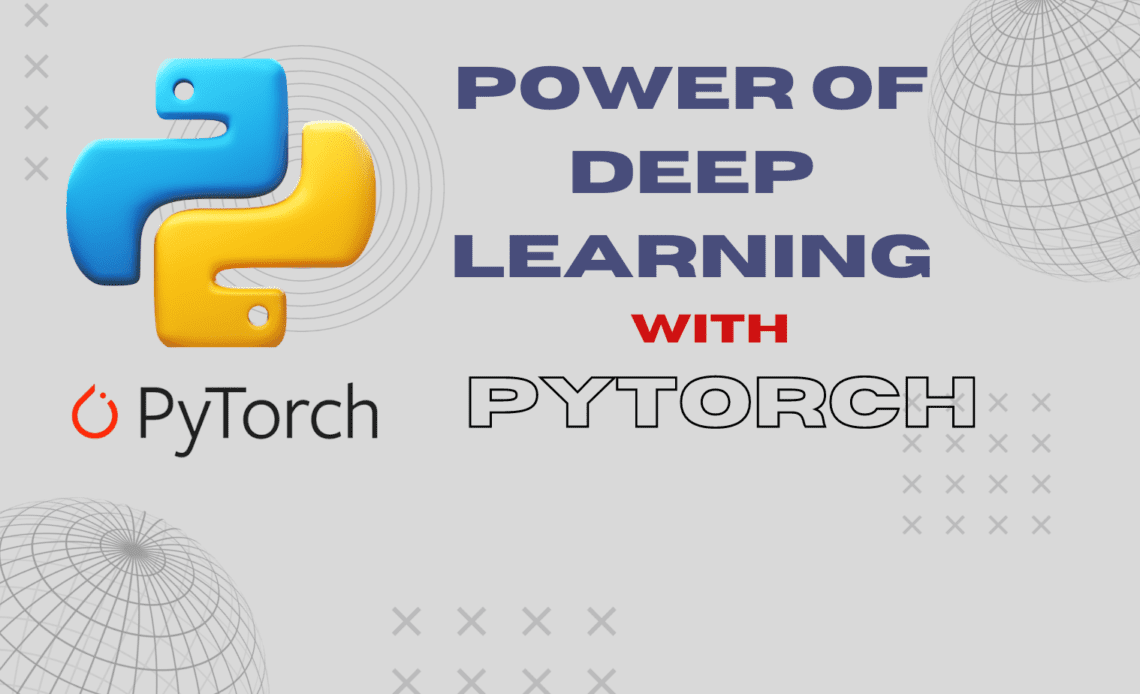
PyTorch: A Brief Overview
PyTorch, an open-source machine learning library, has gained immense popularity for its flexibility and dynamic computation graph, making it a preferred choice for researchers and developers in deep learning. In this blog post, we will embark on a journey to explore the key features of PyTorch and demonstrate its application through a practical example using the MNIST dataset.
The MNIST Dataset: A Gateway to Computer Vision
Before delving into PyTorch specifics, let’s understand the significance of the MNIST dataset. MNIST, short for Modified National Institute of Standards and Technology, is a collection of handwritten digits widely used as a benchmark in the field of computer vision. Comprising 60,000 training images and 10,000 testing images, MNIST serves as a stepping stone for aspiring deep learning practitioners to experiment with image classification.
Crafting a Neural Network: Unveiling the Architecture
To tackle the MNIST challenge, we’ll construct a simple neural network using PyTorch. Our model, named SimpleNN, consists of a flattening layer, two fully connected layers, ReLU activation, and a softmax layer for classification. The architecture is tailored to map the 28×28 pixel input images to the 10 digit classes present in the MNIST dataset.
# Code snippet for defining SimpleNN architecture
import torch
import torch.nn as nn
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.flatten = nn.Flatten()
self.fc1 = nn.Linear(28 * 28, 128)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(128, 10)
self.softmax = nn.Softmax(dim=1)
def forward(self, x):
x = self.flatten(x)
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
x = self.softmax(x)
return x
Preparing Data with PyTorch’s Data Utilities
PyTorch simplifies the process of loading and preprocessing data through its powerful data utilities. Using the torchvision library, we can effortlessly fetch the MNIST dataset and apply transformations such as normalization and tensor conversion.
# Code snippet for loading and preprocessing MNIST data
import torchvision.transforms as transforms
from torchvision.datasets import MNIST
from torch.utils.data import DataLoader
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
train_dataset = MNIST(root='./data', train=True, transform=transform, download=True)
test_dataset = MNIST(root='./data', train=False, transform=transform, download=True)
batch_size = 64
train_loader = DataLoader(dataset=train_dataset, batch_size=batch_size, shuffle=True)
test_loader = DataLoader(dataset=test_dataset, batch_size=batch_size, shuffle=False)
Training and Evaluation: Unveiling the Model’s Performance
With the data prepared, we can move on to training our SimpleNN model. Utilizing a cross-entropy loss and the Adam optimizer, the training loop updates the model’s parameters over multiple epochs. The model is then evaluated on the test set, and its accuracy is calculated.
# Code snippet for training and evaluating the model
import torch.optim as optim
from tqdm import tqdm
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
epochs = 5
for epoch in range(epochs):
running_loss = 0.0
for images, labels in tqdm(train_loader, desc=f'Epoch {epoch + 1}/{epochs}', leave=False):
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f'Training Loss: {running_loss / len(train_loader)}')
# Model evaluation
model.eval()
correct = 0
total = 0
with torch.no_grad():
for images, labels in tqdm(test_loader, desc='Testing', leave=False):
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = correct / total
print(f'Test Accuracy: {accuracy * 100:.2f}%')
Fine-Tuning and Hyperparameter Experimentation
As we conclude our journey, it’s essential to highlight the iterative nature of deep learning. Fine-tuning and experimentation with hyperparameters play a crucial role in enhancing model performance. Adjusting learning rates, layer sizes, or adding regularization techniques are common strategies to refine your model.
In summary, PyTorch provides a robust platform for developing, training and experimenting with deep learning models. The MNIST example showcased here serves as a starting point for your exploration into the world of PyTorch, encouraging you to experiment, iterate, and unlock the full potential of deep learning. Happy coding!