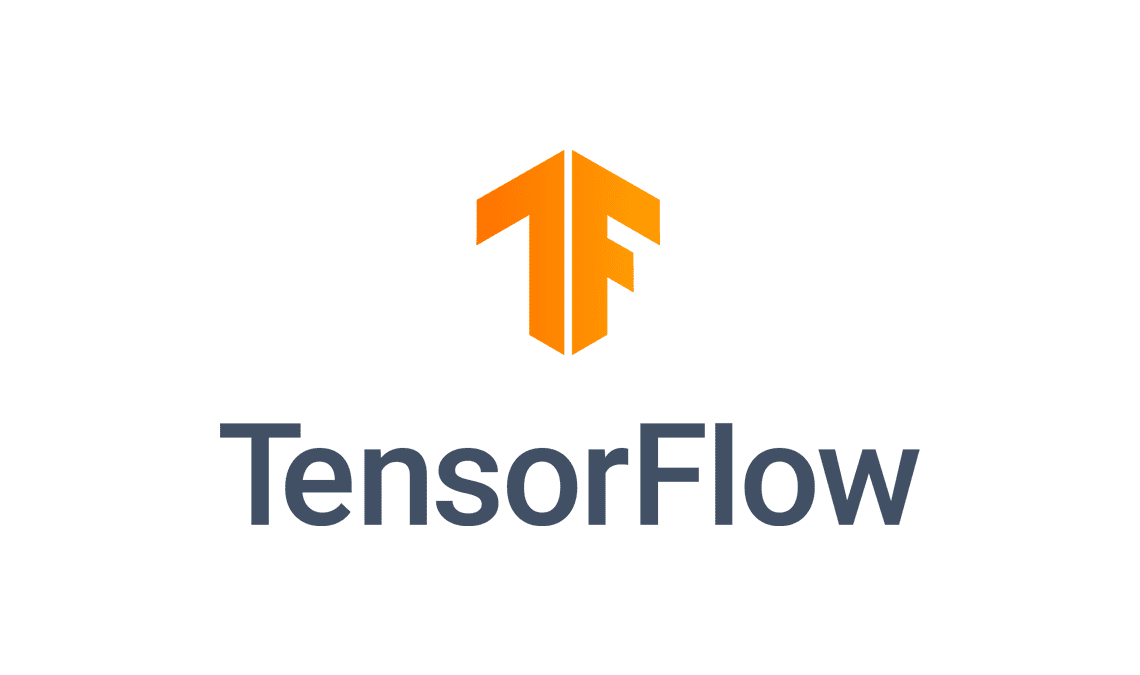
What is TensorFlow?
TensorFlow, developed by the Google Brain team, is an open-source machine learning library that simplifies the process of developing and training deep learning models. It provides a comprehensive platform for building and deploying machine learning applications across a variety of domains.
Key Features of TensorFlow:
- Flexibility: TensorFlow supports both high-level APIs like Keras for quick model development and low-level APIs for fine-tuning and customization.
- Scalability: It allows seamless transition from prototyping on a local machine to training models on distributed systems.
- Community Support: TensorFlow boasts a large and active community, making it easy to find resources, tutorials, and solutions to common problems.
- TensorBoard: A powerful visualization tool that helps in understanding and optimizing the performance of machine learning models.
Now, let’s delve into an example that demonstrates TensorFlow’s capabilities using a classic dataset: MNIST.
MNIST Dataset: A Cornerstone in Computer Vision
Understanding MNIST:
MNIST, short for Modified National Institute of Standards and Technology, is a dataset consisting of 28×28 pixel grayscale images of handwritten digits (0 through 9). It serves as a benchmark in the machine learning community and is often the first dataset developers use to test and validate their models.
Why MNIST?
- Simplicity: The dataset’s simplicity allows for quick experimentation with various machine learning models.
- Benchmarking: Many researchers and practitioners use MNIST as a benchmark to compare the performance of different algorithms.
- Introduction to Image Classification: MNIST is an excellent starting point for learning image classification, a fundamental task in computer vision.
Neural Network Architecture: Building Blocks of Deep Learning
Components of a Neural Network:
- Input Layer: The initial layer that receives the input data.
- Hidden Layers: Layers between the input and output layers where the model learns patterns.
- Output Layer: The final layer that produces the model’s predictions.
Activation Functions:
Activation functions, such as ReLU (Rectified Linear Unit), introduce non-linearity to the model, enabling it to learn complex patterns.
Loss Function and Optimizer:
The loss function measures the model’s performance, and the optimizer adjusts the model’s weights to minimize this loss.
Loading and Preprocessing Data with TensorFlow
Data Utilities in TensorFlow:
TensorFlow provides efficient tools for loading and preprocessing data. The following code snippet demonstrates how to load and normalize the MNIST dataset:
import tensorflow as tf
from tensorflow.keras.datasets import mnist
# Load and preprocess the MNIST dataset
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
train_images, test_images = train_images / 255.0, test_images / 255.0
Training and Evaluating the Model
Model Compilation and Training:
Now, let’s build a simple neural network and train it using TensorFlow:
from tensorflow.keras import layers, models
# Build the neural network model
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)),
layers.Dense(128, activation='relu'),
layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=5, validation_data=(test_images, test_labels))
Evaluating Model Performance:
After training the model, we can evaluate its performance on the test set:
# Evaluate the model
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f"\nTest accuracy: {test_acc}")
Fine-Tuning and Hyperparameter Experimentation
Optimizing Model Performance:
To enhance model performance, fine-tuning and hyperparameter experimentation are crucial. Adjusting parameters like learning rate, batch size, and the number of layers can significantly impact a model’s effectiveness.
Iterative Experimentation:
Consider creating a grid or random search for hyperparameter tuning. TensorFlow’s TensorBoard can assist in visualizing the effects of different configurations on the model’s performance.
# Example of hyperparameter experimentation
from tensorflow.keras.callbacks import TensorBoard
# Set up TensorBoard for visualization
tensorboard_callback = TensorBoard(log_dir='./logs')
# Train the model with TensorBoard callback
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels), callbacks=[tensorboard_callback])
In conclusion, TensorFlow empowers developers and researchers to explore the vast landscape of deep learning. By understanding the basics, leveraging powerful datasets like MNIST, and experimenting with neural network architectures and hyperparameters, you can unlock the full potential of TensorFlow in your machine learning endeavors. Happy coding!