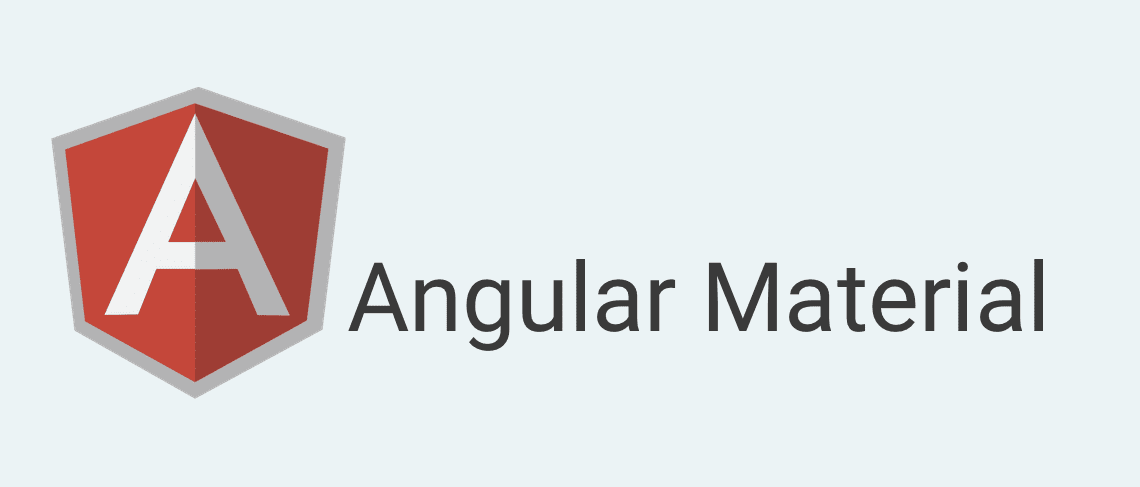
Here is a step-by-step guide on how to build a simple blog using Angular:
- Create a new Angular project using the Angular CLI by running the following command in your terminal:
ng new blog
- Install the Angular Material library, which provides pre-built UI components for your app:
ng add @angular/material
- Create a new component for the blog home page by running the following command in your terminal:
ng generate component home
- In the
home.component.html
file, add a header and a list of blog posts:
<header>
<h1>Welcome to my blog</h1>
</header>
<main>
<ul>
<li *ngFor="let post of blogPosts">
<h2>{{ post.title }}</h2>
<p>{{ post.content }}</p>
</li>
</ul>
</main>
- In the
home.component.ts
file, add an array of blog posts:
import { Component } from '@angular/core';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent {
blogPosts = [
{
title: 'My First Blog Post',
content: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.'
},
{
title: 'My Second Blog Post',
content: 'Sed ut perspiciatis unde omnis iste natus error sit voluptatem.'
},
{
title: 'My Third Blog Post',
content: 'At vero eos et accusamus et iusto odio dignissimos ducimus.'
}
];
}
6. Add the HomeComponent
to the app.component.html
file:
<app-home></app-home>
- Run the app by running the following command in your terminal:
ng serve
This will start a local server, and you can view your blog at http://localhost:4200
.
Congratulations! You’ve just created a simple blog using Angular. Of course, this is just a starting point, and you can customize the blog by adding more components, pages, and functionality.