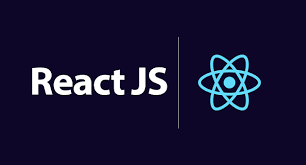
Here is a step by step guide in this ReactJS Tutorial to start with the first react application.
1. To start with ReactJS, we need to first import the react packages as follows.
import React from 'react';
import ReactDOM from 'react-dom';
2. Save the file as index.js in src/ folder
We will write a simple code in this tutorial React JS, wherein we will display the message “Hello, from onlinelearningportal.website!”
ReactDOM.render(
<h1>Hello, from onlinelearningportal.website!</h1>,
document.getElementById('root')
);
ReactDOM.render will add the <h1> tag to the element with id root. Here is the html file we are having:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>ReactJS Demo</title>
</head>
<body>
<div id = "root"></div>
</body>
</html>
Next in this React.js Tutorial, we need to compile the code to get the output in the browser.
Here is the folder structure:
reactproj/
node_modules/
src/
index.js
package.json
public/
index.html
We have added the commands to compile the final file in package.json as follows:
"scripts": { "start": "react-scripts start" },
To compile the final file run following command:
npm run start
When you run above command, it will compile the files and notify you if any error, if all looks good, it will open the browser and the run the index.html file at http://localhost:3000/index.html
Command: npm run start:
C:\reactproj>npm run start
> reactproj@1.0.0 start C:\reactproj
> react-scripts start
The URL http://localhost:3000 will open in the browser once the code is compiled as shown below: