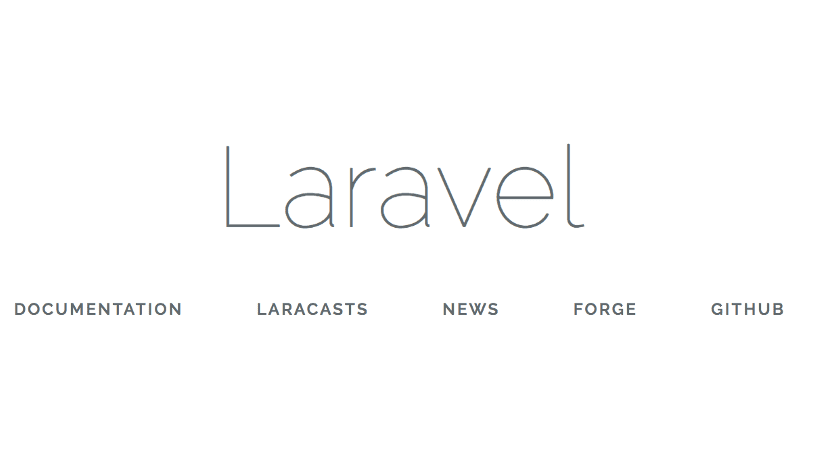
What is Laravel?
Laravel is an MIT license open source “PHP framework” based on the MVC design pattern. It is created by Taylor Otwell. Laravel provides expressive and elegant syntax that helps in creating a wonderful web application easily and quickly. Due to MIT’s license, its source code is hosted on GitHub. It is a reliable PHP framework as it follows expressive and accurate language rules.
What is Composer?
It is an application-level package manager for PHP. It provides a standard format for managing PHP software dependencies and libraries.
What is HTTP middleware?
HTTP middleware is a technique for filtering HTTP requests. Laravel includes a middleware that checks whether the application user is authenticated or not.
To create a new middleware, use the make:middleware
Artisan command:
php artisan make:middleware MiddlewareName
What are Events in Laravel?
An event is an action or occurrence recognized by a program that may be handled by the program or code. Laravel events provide a simple observer implementation, that allowing you to subscribe and listen for various events/actions that occur in your application.
All Event classes are generally stored in the app/Events directory, while their listeners are stored in the app/Listeners of your application.
What are the validations in Laravel?
Validations are approaches that Laravel uses to validate the incoming data within the application.
They are a handy way to ensure that data is in a clean and expected format before it gets entered into the database. Laravel consists of several different ways to validate the incoming data of the application. By default, the base controller class of Laravel uses a ValidatesRequests trait to validate all the incoming HTTP requests with the help of powerful validation rules.
Laravel validation Example:-
$validate_data = $request->validate([
'name' => 'required|max:255',
'user_name' => 'required|alpha_num|max:255',
'user_age' => 'required|numeric',
]);
What are the main features of Laravel?
Some of the main features of Laravel are:-
- Eloquent ORM
- Query builder
- Reverse Routing
- Restful Controllers
- Migrations
- Database Seeding
- Unit Testing
- Homestead
How to install Laravel via composer?
You can install Laravel via composer by running the below command.
composer create-project laravel/laravel
application-name
What is Eloquent ORM?
Eloquent ORM (Object-Relational Mapping) is one of the main features of the Laravel framework. It may be defined as an advanced PHP implementation of the active record pattern.
Active record pattern is an architectural pattern that is found in software. It is responsible for keeping in-memory object data in relational databases
Eloquent ORM is also responsible for providing the internal methods at the same time when enforcing constraints on the relationship between database objects. Eloquent ORM represents database tables as classes, with their object instances tied to single table rows while following the active record pattern.
What is Query Builder in Laravel?
Laravel’s Query Builder provides more direct access to the database, an alternative to the Eloquent ORM. It doesn’t require SQL queries to be written directly. Instead, it offers a set of classes and methods which are capable of building queries programmatically. It also allows specific caching of the results of the executed queries.
How to clear cache in Laravel?
The syntax to clear cache in Laravel is given below:
- php artisan cache:clear
- php artisan config:clear
- php artisan optimize:clear
Name aggregates methods of the query builder.
Aggregates methods of query builder are:
1) max()
2) min()
3) sum()
4) avg()
5) count()
What is a Route?
A route is basically an endpoint specified by a URI (Uniform Resource Identifier). It acts as a pointer in the Laravel application.
Most commonly, a route simply points to a method on a controller and also dictates which HTTP methods are able to hit that URI.
Why use Route?
Routes are stored inside files under the /routes folder inside the project’s root directory. By default, there are a few different files corresponding to the different “sides” of the application (“sides” come from the hexagonal architecture methodology).
Explain Migrations in Laravel
Laravel Migrations are like version control for the database, allowing a team to easily modify and share the application’s database schema. Migrations are typically paired with Laravel’s schema builder to easily build the application’s database schema.
How to put Laravel applications in maintenance mode?
Maintenance mode is used to put a maintenance page to customers and under the hood, we can do software updates, bug fixes, etc. Laravel applications can be put into maintenance mode using the below command:
php artisan down
And can put the application again on live using the below command:
php artisan up
Also, it is possible to access the website in maintenance mode by whitelisting particular IPs.
What are the default route files in Laravel?
Below are the four default route files in the routes folder in Laravel:
- web.php – For registering web routes.
- api.php – For registering API routes.
- console.php – For registering closure-based console commands.
- channel.php – For registering all your event broadcasting channels that your application supports.
What are seeders in Laravel?
Seeders in Laravel are used to put data in the database tables automatically. After running migrations to create the tables, we can run `php artisan db:seed` to run the seeder to populate the database tables.
We can create a new Seeder using the below artisan command:
php artisan make:seeder [className]
It will create a new Seeder like below:
<?php
use App\Models\Auth\User;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Seeder;
class UserTableSeeder extends Seeder
{
/**
* Run the database seeds.
*/
public function run()
{
factory(User::class, 10)->create();
}
}
The run() method in the above code snippet will create 10 new users using the User factory.
Factories will be explained in the next question.
What are factories in Laravel?
Factories are a way to put values in the fields of a particular model automatically. Like, for testing when we add multiple fake records in the database, we can use factories to generate a class for each model and put data in fields accordingly. Every new Laravel application comes with database/factories/UserFactory.php which looks like below:
<?php
namespace Database\Factories;
use App\Models\User;
use Illuminate\Database\Eloquent\Factories\Factory;
use Illuminate\Support\Str;
class UserFactory extends Factory
{
/**
* The name of the factory's corresponding model.
*
* @var string
*/
protected $model = User::class;
/**
* Define the model's default state.
*
* @return array
*/
public function definition()
{
return [
'name' => $this->faker->name,
'email' => $this->faker->unique()->safeEmail,
'email_verified_at' => now(),
'password' => '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', // password
'remember_token' => Str::random(10),
];
}
}
We can create a new factory using php artisan make:factory UserFactory --class=User
.
The above command will create a new factory class for the User model. It is just a class that extends the base Factory class and makes use of the Faker class to generate fake data for each column. With the combination of factory and seeders, we can easily add fake data into the database for testing purposes.
What is Localization in Laravel?
Localization is a way to serve content concerning the client’s language preference. We can create different localization files and use a laravel helper method like this: `__(‘auth.error’)` to retrieve translation in the current locale. These localization files are located in the resources/lang/[language] folder.
What are Requests in Laravel?
Requests in Laravel are a way to interact with incoming HTTP requests along with sessions, cookies, and even files if submitted with the request.
The class responsible for doing this is Illuminate\Http\Request.
When any request is submitted to a laravel route, it goes through to the controller method, and with the help of dependency Injection, the request object is available within the method. We can do all kinds of things with the request like validating or authorizing the request, etc.
How to pass CSRF token with ajax request?
In between head, tag put <meta name=”csrf-token” content=”{{ csrf_token() }}”> and in Ajax, we have to add
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
How to do request validation in Laravel?
Request validation in laravel can be done with the controller method or we can create a request validation class that holds the rules of validation and the error messages associated with it.
One example of it can be seen below:
/**
* Store a new blog post.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$validated = $request->validate([
'title' => 'required|unique:posts|max:255',
'body' => 'required',
]);
// The blog post is valid...
}