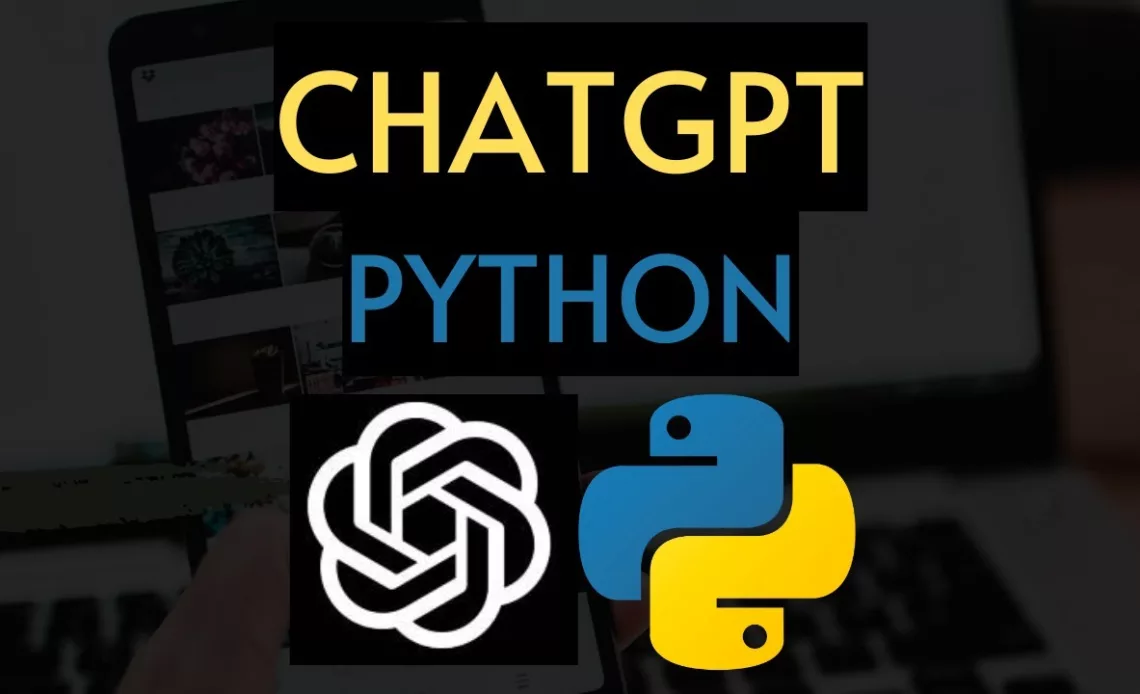
Let’s walk through a simple example of using Python with ChatGPT. We’ll create a program that generates text based on a prompt provided by the user.
To get started, we’ll need to install the openai
Python package, which provides a simple interface for accessing the ChatGPT API. You can install it using pip:
pip install openai
Next, we’ll need to set up an OpenAI API key, which you can get by signing up for an OpenAI account. Once you have your API key, you can set it as an environment variable in your terminal:
export OPENAI_API_KEY=your-api-key-here
Now, we’re ready to create our program. Here’s the code:
import openai
import os
openai.api_key = os.environ["OPENAI_API_KEY"]
def generate_text(prompt):
response = openai.Completion.create(
engine="davinci",
prompt=prompt,
max_tokens=1024,
n=1,
stop=None,
temperature=0.5,
)
return response.choices[0].text
prompt = input("Enter a prompt: ")
generated_text = generate_text(prompt)
print(generated_text)
Let’s walk through the code. First, we import the openai
package and set the API key using the os
module to access the environment variable. Then, we define a function called generate_text
that takes a prompt
parameter.
Inside the generate_text
function, we use the openai.Completion.create
method to generate text based on the prompt. We specify the engine
as “davinci”, which is the most powerful and capable model provided by OpenAI. We also set the max_tokens
to 1024, which limits the length of the generated text. Finally, we set the temperature
to 0.5, which controls the “creativity” of the model – lower temperatures will generate more predictable text, while higher temperatures will generate more varied text.
Once we have our generated text, we return the first choice (there is only one in this case).
In the main part of the program, we prompt the user to enter a prompt, then call the generate_text
function with the prompt. Finally, we print out the generated text.
That’s it! With just a few lines of code, we’ve created a simple program that can generate natural language text based on a prompt. Of course, this is just the beginning – you can use ChatGPT for a wide range of applications, from chatbots to content generation to language translation and more.