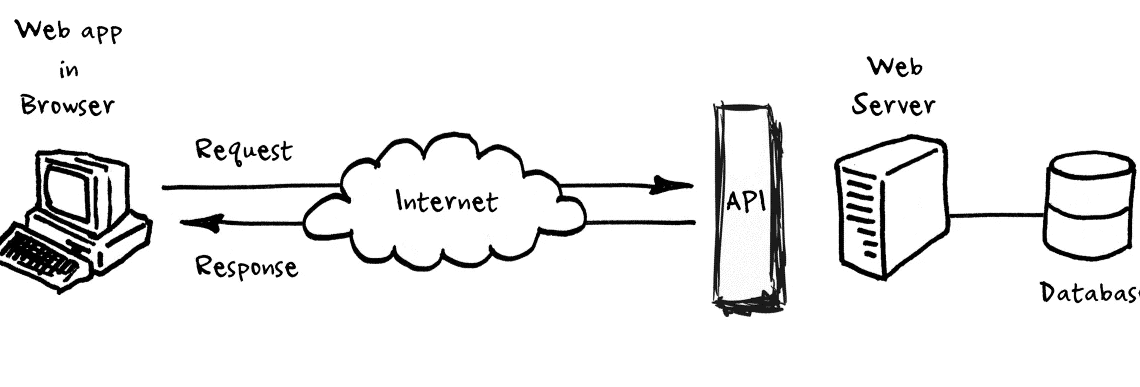
Introduction: Python has long been a popular programming language, known for its simplicity and versatility. While it has been extensively used for a wide range of applications, including data science and automation, Python’s potential in web development has gained significant attention in recent times. In this article, we delve into the rising star of the Python web development ecosystem: FastAPI. We explore why FastAPI has garnered immense popularity among developers and how it revolutionizes the way we build web applications.
Content:
- Understanding the Python Web Development Landscape:
- Brief overview of popular Python web frameworks (Django, Flask, etc.)
- Advantages and limitations of existing frameworks
Python Example Code:
# Flask example
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return 'Hello, Flask!'
if __name__ == '__main__':
app.run()
# Django example
from django.http import HttpResponse
from django.urls import path
def hello(request):
return HttpResponse("Hello, Django!")
urlpatterns = [
path('', hello),
]
- Introducing FastAPI:
- What is FastAPI and how does it differ from traditional frameworks?
- Key features that make FastAPI stand out
- Performance benchmarks and scalability advantages
Python Example Code:
from fastapi import FastAPI
app = FastAPI()
@app.get('/')
def hello():
return {'message': 'Hello, FastAPI!'}
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
- Rapid Development with FastAPI:
- Leveraging the power of type hints and automatic API documentation
- Asynchronous programming with FastAPI and its benefits
- Integration with popular databases and third-party libraries
Python Example Code:
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
price: float
@app.post('/items/')
def create_item(item: Item):
# Save item to database
return {'message': 'Item created successfully'}
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
- Exploring FastAPI’s Ecosystem:
- Authentication and authorization in FastAPI
- Testing and debugging with FastAPI
- Building real-time applications with WebSockets
Python Example Code:
from fastapi import FastAPI, WebSocket
app = FastAPI()
@app.websocket('/ws/')
async def websocket_endpoint(websocket: WebSocket):
await websocket.accept()
while True:
data = await websocket.receive_text()
# Process incoming data
await websocket.send_text('Response: ' + data)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
- Deployment and Production-Ready Applications:
- Containerization and deployment options (Docker, Kubernetes)
- Handling security concerns and best practices
- Monitoring and scaling FastAPI applications
Conclusion: FastAPI has emerged as a powerful and efficient framework for web development in Python, providing developers with a high-performance option that is easy to use and maintain. Its ability to handle asynchronous tasks, seamless integration with modern technologies, and emphasis on type safety make it a compelling choice for building robust and scalable web applications. As Python continues to dominate various domains, FastAPI stands as a testament to its adaptability in the ever-evolving world of web development.