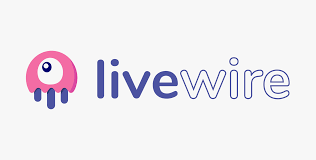
In today’s web development landscape, users expect interactive and dynamic interfaces that update in real-time without page refreshes. Laravel Livewire is a powerful tool that allows you to build such interfaces using Laravel’s familiar syntax. In this blog post, we will dive into the world of Livewire and explore how to create real-time dynamic interfaces with ease.
What is Laravel Livewire? Before we dive into the implementation details, let’s quickly understand what Laravel Livewire is. Livewire is a full-stack framework for Laravel that enables developers to build dynamic interfaces using Laravel’s server-side rendering capabilities. With Livewire, you can write interactive components using PHP and Blade templates, without the need for writing JavaScript code manually.
Setting Up Livewire: To get started with Livewire, make sure you have Laravel installed. If you haven’t already, create a new Laravel project using composer create-project --prefer-dist laravel/laravel blog
. Once your project is set up, you can install Livewire by running composer require livewire/livewire
.
Creating a Real-Time Dynamic Interface: Let’s start by building a simple example of a real-time dynamic interface—a live comment section.
- Set up the Route: In your
web.php
file, define a route that points to a Livewire component:
use App\Http\Livewire\CommentSection;
Route::get('/comments', CommentSection::class);
- Create the Livewire Component: Generate a Livewire component called
CommentSection
using the following command:php artisan livewire:make CommentSection
.
Open the newly generated CommentSection
component file and modify the render
method as follows:
public function render()
{
$comments = Comment::orderBy('created_at', 'desc')->get();
return view('livewire.comment-section', [
'comments' => $comments,
]);
}
- Create the Blade Template: Create a Blade template file called
comment-section.blade.php
in theresources/views/livewire
directory. Use the following code as a starting point:
<div>
<h2>Live Comment Section</h2>
<ul>
@foreach($comments as $comment)
<li>{{ $comment->content }}</li>
@endforeach
</ul>
<input type="text" wire:model="newComment">
<button wire:click="addComment">Add Comment</button>
</div>
- Implement Dynamic Behavior: Open the
CommentSection
component file again and add the following methods:
public $newComment;
public function addComment()
{
Comment::create([
'content' => $this->newComment,
]);
$this->newComment = '';
$this->render();
}
Conclusion: Congratulations! You have successfully built a real-time dynamic interface using Laravel Livewire. Livewire simplifies the process of creating interactive components without the need for writing JavaScript code. Experiment with Livewire’s capabilities to build even more engaging and real-time interfaces in your Laravel applications.
Feel free to explore more features offered by Livewire, such as form validation, events, and data binding, to enhance the interactivity of your web applications.
That’s it for this blog post. Happy coding with Laravel Livewire!