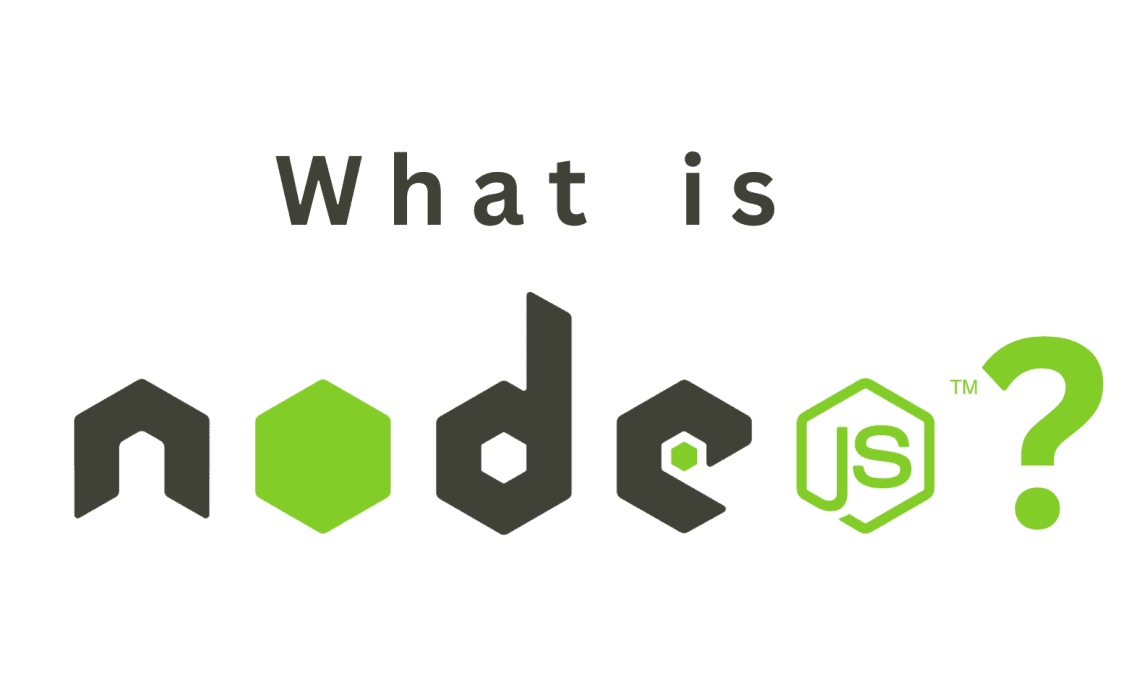
Node.js is a powerful runtime environment for executing JavaScript code outside of a web browser. It offers various features and modules that enable developers to build robust and scalable applications. In this article, we’ll explore the different types of modules available in Node.js and how they can be used to enhance your applications.
Global Features in Node.js
Node.js provides a set of global features that are readily available in any application without the need for explicit imports. These features include keywords like const
and function
that are fundamental to JavaScript. Additionally, Node.js offers access to certain global objects such as process
, which provides information and control over the current Node.js process.
Global features are always accessible within your Node.js files, allowing you to use them directly without any additional setup. This accessibility makes them convenient for performing common tasks and interacting with the underlying system.
Core Node.js Modules
Core Node.js modules are pre-installed modules that come bundled with the Node.js runtime. These modules provide essential functionality for various tasks, ranging from file system operations to network communication.
Some examples of core modules in Node.js include:
- File System (fs): The
fs
module enables interaction with the file system, allowing you to read, write, and manipulate files. - Path (path): The
path
module provides utilities for working with file and directory paths, making it easier to handle file paths in a cross-platform manner. - HTTP (http): The
http
module offers a set of classes and methods to create HTTP servers and make HTTP requests.
Core Node.js modules don’t require any additional installation. However, to use their features, you need to import them into your application using the require
keyword. For example, to use the file system module, you would write:
const fs = require('fs');
Once imported, you can utilize the functions and objects provided by the core module in your code.
Third-Party Modules with npm
In addition to the core modules, Node.js allows you to extend its capabilities by utilizing third-party modules. These modules are created by the Node.js community and can be installed using the Node Package Manager (npm). npm provides a vast collection of modules, offering a wide range of functionalities that can be seamlessly integrated into your applications.
To install a third-party module, you can use the following command in your terminal or command prompt:
npm install --save <module-name>
Once the module is installed, you can import it into your Node.js files similar to core modules. This import step ensures that your application can access the features provided by the third-party module. For example, if you want to use the express-session
module, you would write:
const sessions = require('express-session');
Third-party modules greatly expand the capabilities of Node.js, allowing you to incorporate advanced functionalities such as web frameworks, database connectors, authentication systems, and more.
Conclusion
Understanding the different types of modules in Node.js is crucial for developing efficient and feature-rich applications. Global features, core modules, and third-party modules each play a significant role in extending the capabilities of Node.js.
Global features provide fundamental JavaScript elements that are readily accessible within your application. Core modules, on the other hand, offer essential functionalities without requiring additional installations. Lastly, third-party modules, installed via npm, unlock a vast ecosystem of pre-built solutions to enhance your Node.