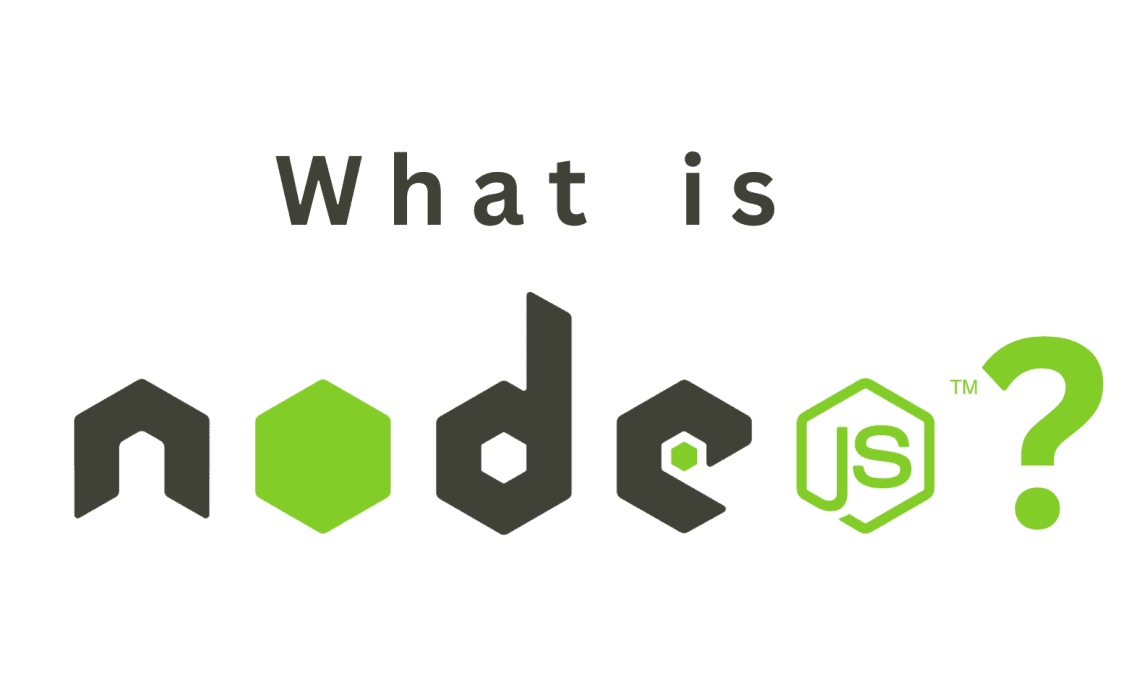
Introduction:
In this tutorial, we will learn how to build a simple Node.js server using Express, a popular web application framework for Node.js. We will cover the basics of setting up the server, handling routes, and serving static files. Additionally, we will explore how to use templates to create dynamic web pages. Let’s get started!
Setting up the Server:
To begin, make sure you have Node.js installed on your machine. Create a new directory for your project and navigate to it in your terminal. Run the following command to initialize a new Node.js project:
npm init -y
Next, install Express by running the following command:
npm install express
Now, let’s create a file named app.js
and open it in your preferred code editor. Import the Express module and create a new instance of the Express application:
const express = require('express');
const app = express();
Handling Routes:
To handle routes in Express, you can use the app.get()
method. Let’s create a simple route that responds with “Hello, World!” when the root URL is accessed:
app.get('/', (req, res) => {
res.send('Hello, World!');
});
Serving Static Files:
To serve static files, such as CSS, JavaScript, or images, we can use the express.static()
middleware provided by Express. Create a new directory named public
in your project’s root folder. Place your static files inside this directory. To serve the files, add the following code:
app.use(express.static('public'));
Now, any files placed inside the public
directory can be accessed directly by the client.
Templates for Dynamic Pages:
To create dynamic web pages, we can use templates. There are several template engines available for Express, but for this example, we will use EJS (Embedded JavaScript). First, install EJS by running:
npm install ejs
Next, create a new directory named views
in your project’s root folder. Inside the views
directory, create a file named index.ejs
. This will be our template file. Here’s an example of an index.ejs
file:
<!DOCTYPE html>
<html>
<head>
<title>My Express App</title>
<link rel="stylesheet" href="/css/style.css">
</head>
<body>
<h1>Welcome to My Express App!</h1>
<p><%= message %></p>
</body>
</html>
In the app.js
file, set the view engine to EJS and render the index.ejs
template:
app.set('view engine', 'ejs');
app.get('/', (req, res) => {
const message = 'This is a dynamic message!';
res.render('index', { message });
});
Conclusion:
In this tutorial, we learned how to build a simple Node.js server using Express. We covered setting up the server, handling routes, serving static files, and using templates for dynamic web pages. With this foundation, you can now start building more complex web applications with Node.js and Express.
We hope you found this tutorial helpful. Happy coding!