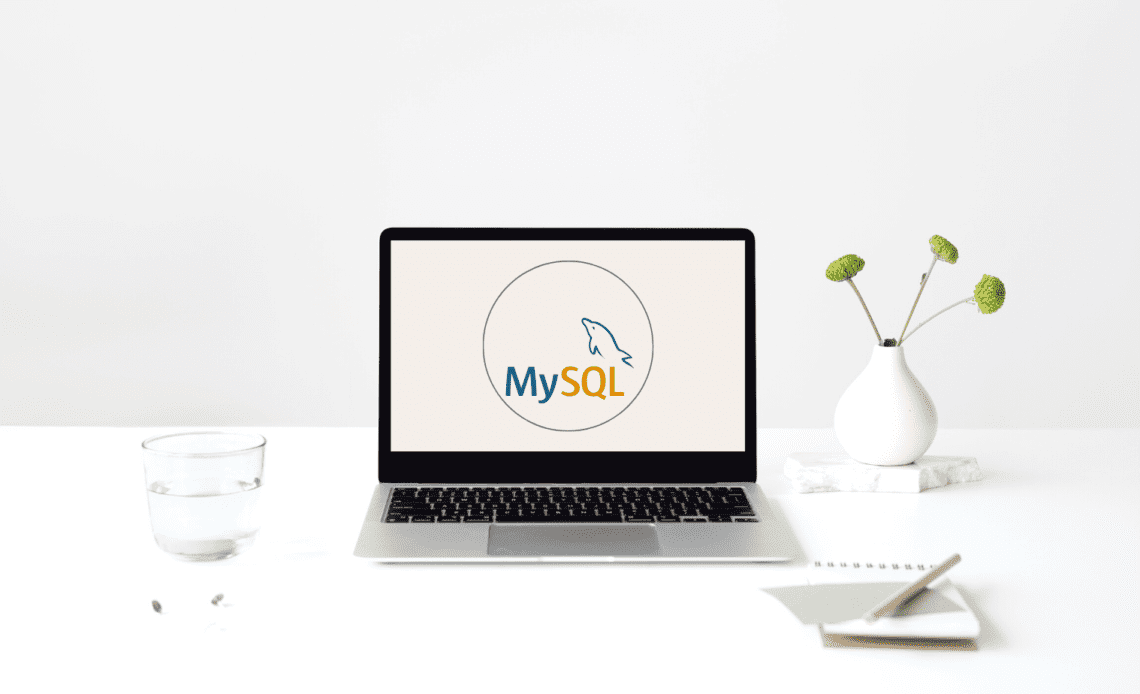
Introduction: MySQL is a popular open-source relational database management system (RDBMS) used to store and manage vast amounts of data. Whether you’re a beginner or an experienced developer, understanding the fundamentals of MySQL is essential for efficient database operations.
What is MySQL? MySQL is a relational database management system that allows you to store and retrieve data in a structured manner. It uses a client-server architecture and is known for its speed, reliability, and scalability.
Database Definitions:
- Database: A database is a structured collection of data organized into tables, making it easy to store and retrieve information efficiently.
- Table: A table is a fundamental unit of data storage in MySQL, consisting of rows and columns, similar to a spreadsheet.
- Column: Columns define the type of data that can be stored in a table, such as integers, text, or dates.
- Row: Rows contain the actual data in a table, with each row representing a unique record.
Keys in MySQL:
In MySQL, a key is a field or a set of fields that are used to identify and establish relationships between rows in a table. Keys play a pivotal role in ensuring data integrity, optimizing query performance, and connecting tables within a relational database.
Types of Keys in MySQL:
Primary Key:
- A primary key uniquely identifies each row in a table.
- It enforces data integrity by ensuring that no duplicate values exist.
- Here’s an example of defining a primary key in a
users
table:
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50),
email VARCHAR(100)
);
Foreign Key:
- A foreign key establishes a link between two tables by referencing the primary key of another table.
- It enforces referential integrity, ensuring that relationships between tables are maintained.
- Example: Linking a
orders
table to acustomers
table using a foreign key.
CREATE TABLE orders (
order_id INT PRIMARY KEY,
customer_id INT,
order_date DATE,
FOREIGN KEY (customer_id) REFERENCES customers(customer_id)
);
Unique Key:
- A unique key ensures that values in a column or a set of columns are unique across the table.
- It’s useful for preventing duplicate data entries.
- Example: Creating a unique key for the
email
column in acustomers
table.
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100) UNIQUE
);
Index:
- An index is a data structure that enhances query performance by speeding up data retrieval.
- It can be created on one or more columns to optimize searches.
- Example: Creating an index on the
last_name
column of anemployees
table.
CREATE INDEX idx_last_name ON employees(last_name);
Certainly! Here’s a blog post about MySQL queries, complete with examples to help readers understand how to retrieve and manipulate data from a MySQL database.
Basics of MySQL Queries:
Before diving into complex queries, let’s cover the fundamental components of MySQL queries:
- SELECT Statement:
- The SELECT statement is used to retrieve data from one or more tables.
- It allows you to specify which columns you want to retrieve and can include filtering conditions.
- Example: Retrieving all employee names and their salaries from an
employees
table.
SELECT employee_name, salary FROM employees;
- INSERT Statement:
- The INSERT statement is used to add new records to a table.
- You specify the table and the values to be inserted into each column.
- Example: Inserting a new employee into the
employees
table.
INSERT INTO employees (employee_name, salary) VALUES ('John Doe', 50000);
- UPDATE Statement:
- The UPDATE statement is used to modify existing records in a table.
- You specify the table, the columns to be updated, and the new values.
- Example: Updating the salary of an employee named ‘John Doe.’
UPDATE employees SET salary = 55000 WHERE employee_name = 'John Doe';
- DELETE Statement:
- The DELETE statement is used to remove records from a table based on specified conditions.
- Be cautious, as it permanently deletes data.
- Example: Deleting an employee record based on their name.
DELETE FROM employees WHERE employee_name = 'John Doe';
Advanced MySQL Queries:
Now, let’s explore some more advanced queries:
- JOIN Clause:
- JOIN allows you to combine data from multiple tables based on related columns.
- Example: Retrieving orders with customer information from
orders
andcustomers
tables.
SELECT orders.order_id, customers.customer_name
FROM orders
JOIN customers ON orders.customer_id = customers.customer_id;
- WHERE Clause:
- The WHERE clause is used to filter rows based on specific conditions.
- Example: Retrieving employees with a salary greater than $60,000.
SELECT employee_name, salary
FROM employees
WHERE salary > 60000;
- GROUP BY and Aggregate Functions:
- GROUP BY groups rows with similar values in a specified column and allows you to apply aggregate functions like SUM, AVG, etc.
- Example: Finding the total salary expenditure for each department.
SELECT department, SUM(salary) AS total_salary
FROM employees
GROUP BY department;
Conclusion:
MySQL is a powerful tool for managing and manipulating data in various applications. Understanding database definitions, keys, and common queries is crucial for anyone working with MySQL. With this knowledge, you can optimize your database design, ensure data integrity, and create efficient data-driven applications.
By incorporating these MySQL fundamentals into your development process, you’ll be better equipped to harness the full potential of this versatile RDBMS.