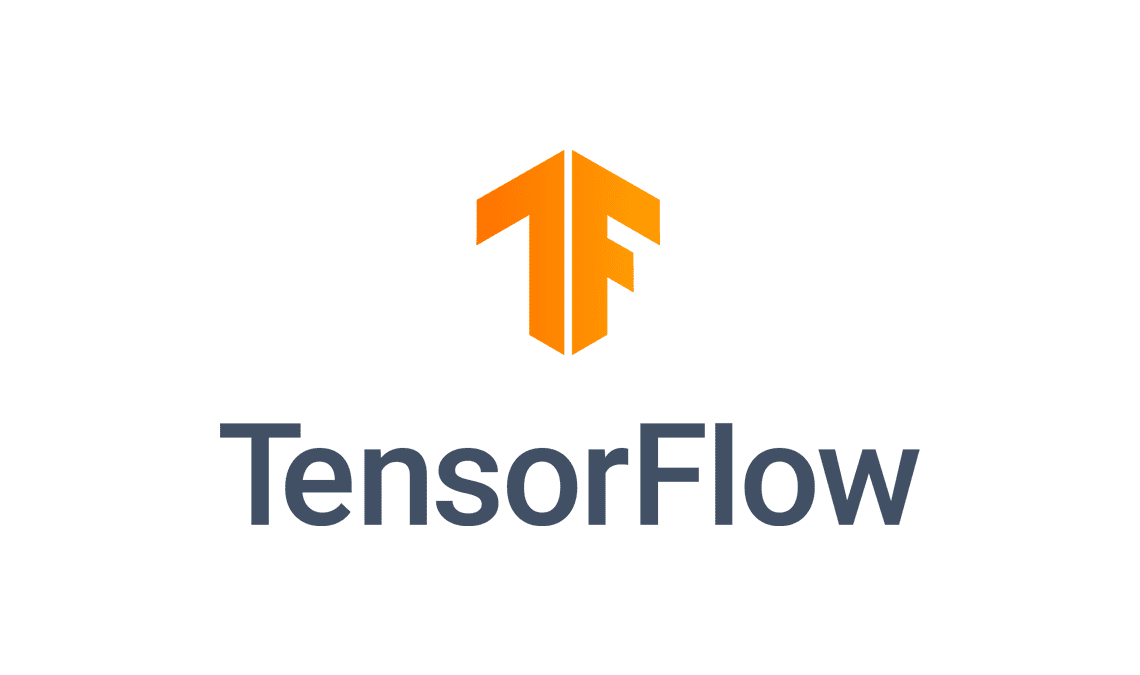
If you’re interested in diving into the exciting world of deep learning and neural networks, TensorFlow is an excellent place to start. TensorFlow is an open-source machine learning framework developed by Google that has gained immense popularity due to its flexibility and ease of use. In this post, we’ll walk you through the basics of TensorFlow and provide a simple example to get you started.
What is TensorFlow?
TensorFlow is a powerful library that allows you to build and train machine learning models, especially deep neural networks, for various tasks like image classification, natural language processing, and more. It was designed with flexibility in mind and can be used for both research and production applications.
Here are some key features of TensorFlow:
- Flexibility: TensorFlow provides a high-level API (Keras) that makes it easy to build and train models quickly, as well as a lower-level API that gives you more control over model architecture and training.
- Scalability: You can use TensorFlow on a range of devices, from CPUs to GPUs and TPUs, making it suitable for both small-scale and large-scale projects.
- Community and Ecosystem: TensorFlow has a large and active community, which means there are plenty of resources, tutorials, and pre-trained models available to help you get started.
Installing TensorFlow
Before we can start using TensorFlow, we need to install it. You can do this easily with pip:
pip install tensorflow
If you have a compatible GPU and want to enable GPU support, you can install TensorFlow with GPU support using:
pip install tensorflow-gpu
A Simple TensorFlow Example
Let’s dive into a simple example to demonstrate how to use TensorFlow. We’ll create a basic neural network to solve a classification problem. In this example, we’ll use the classic Iris dataset, which consists of iris flowers with different species (setosa, versicolor, and virginica) based on petal and sepal measurements.
import tensorflow as tf
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Load the Iris dataset
data = load_iris()
X, y = data.data, data.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the features
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Build a simple neural network
model = Sequential([
Dense(10, activation='relu', input_shape=(4,)),
Dense(3, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=100, verbose=1)
# Evaluate the model
test_loss, test_accuracy = model.evaluate(X_test, y_test)
print(f"Test accuracy: {test_accuracy}")
In this code:
- We load the Iris dataset using scikit-learn.
- We split the dataset into training and testing sets.
- We standardize the features to have zero mean and unit variance, which is a common preprocessing step in machine learning.
- We create a simple neural network using TensorFlow’s Keras API. This network consists of one hidden layer with 10 neurons and ReLU activation, followed by an output layer with 3 neurons (one for each iris species) and softmax activation.
- We compile the model with an optimizer (Adam), a loss function (sparse_categorical_crossentropy, suitable for classification problems), and a metric to measure performance (accuracy).
- We train the model on the training data for 100 epochs.
- Finally, we evaluate the model’s performance on the test data.
Conclusion
TensorFlow is a versatile and powerful tool for building and training machine learning models. This post covered the basics and provided a simple example to help you get started. As you explore further, you’ll discover the vast capabilities of TensorFlow and its potential for tackling a wide range of machine learning tasks. Whether you’re interested in image recognition, natural language processing, or any other machine learning application, TensorFlow is a valuable resource to have in your toolkit.
So, don’t hesitate to dive in, experiment with different models, and explore the TensorFlow ecosystem. Happy coding!