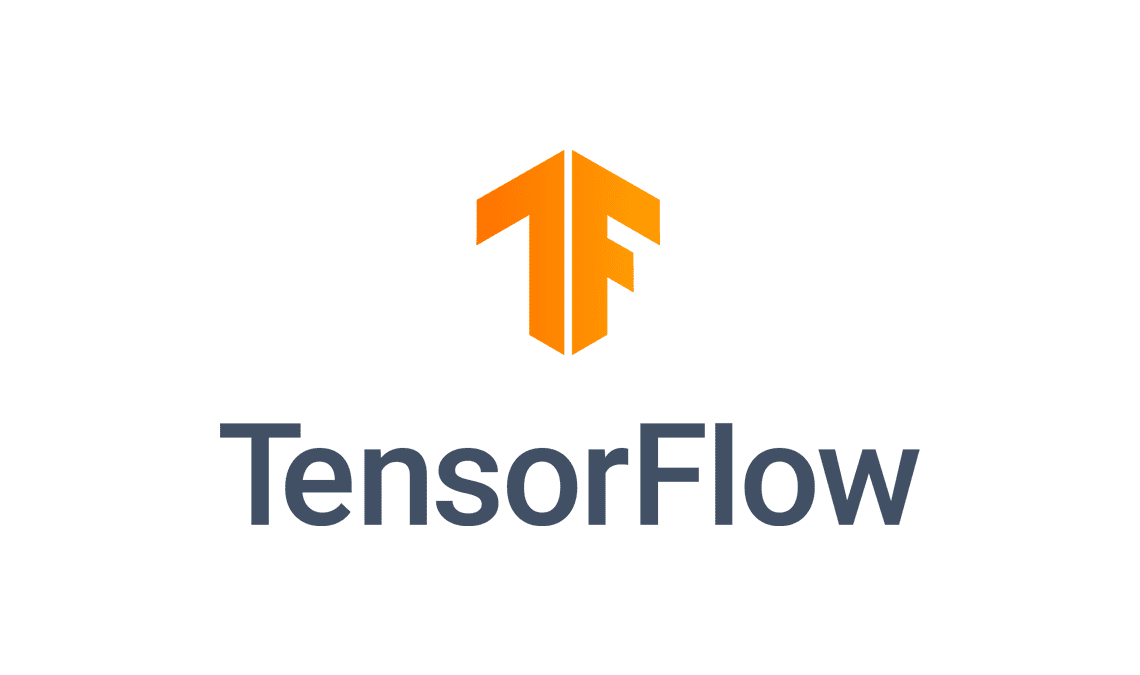
Face recognition is a fascinating application of machine learning and deep learning. With the advent of powerful libraries like TensorFlow, it has become easier than ever to develop robust face recognition systems. In this guide, we’ll walk you through the process of building a simple face recognition system using TensorFlow.
Prerequisites
Before we dive into the code, make sure you have the following prerequisites installed:
- Python: You’ll need Python 3.x installed on your system.
- TensorFlow: Install TensorFlow using
pip
:
pip install tensorflow
- OpenCV: OpenCV is a popular library for computer vision. Install it with
pip
:
pip install opencv-python
- Numpy: This library is used for numerical operations in Python:
pip install numpy
- Pillow: For image processing tasks:
pip install pillow
Face Recognition using TensorFlow
Let’s build a face recognition system that can identify faces in images using TensorFlow. We’ll use a pre-trained model for this example.
import tensorflow as tf
import cv2
import numpy as np
# Load a pre-trained face recognition model
model = tf.keras.applications.MobileNetV2(weights="imagenet")
# Load an image for face recognition
image = cv2.imread("path_to_image.jpg")
# Preprocess the image
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (224, 224))
image = tf.keras.applications.mobilenet_v2.preprocess_input(image)
# Make predictions on the image
predictions = model.predict(np.expand_dims(image, axis=0))
# Get the top prediction
predicted_label = tf.keras.applications.mobilenet_v2.decode_predictions(predictions)[0][0]
# Display the results
print("Predicted Label: {}".format(predicted_label[1]))
In the code above, we load a pre-trained MobileNetV2 model and use it for face recognition. We preprocess the input image, make predictions, and display the top predicted label, which should be a person’s name if the model was trained for face recognition.
Training Your Own Model
For more accurate face recognition, you may want to train your own model. Collect a dataset of face images and use transfer learning to fine-tune a pre-trained model or train a custom deep learning model.
Here’s a simplified example of training a face recognition model:
import tensorflow as tf
from tensorflow.keras import layers, models
# Load your face dataset and labels
X_train, y_train = load_face_dataset()
# Create a deep learning model
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(128, activation='relu'),
layers.Dense(num_classes, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10)
In this example, you should replace load_face_dataset
with your own data loading function. You can use techniques like data augmentation and transfer learning to improve model accuracy.
Conclusion
Face recognition is a powerful application of deep learning and TensorFlow. Whether you use a pre-trained model or train your own, TensorFlow provides a robust platform for building face recognition systems. Experiment with different models and datasets to achieve the best results for your specific use case.
Happy coding and recognition! 📸👤