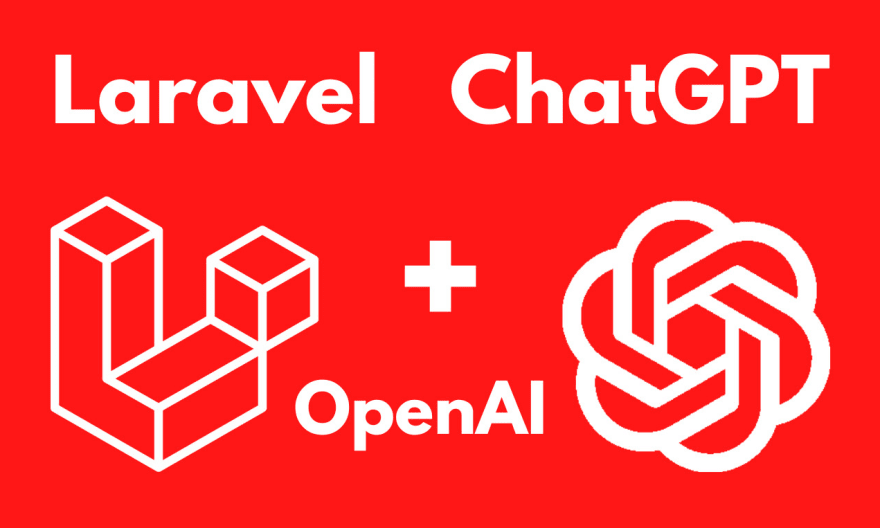
Hello! I’d be happy to help you integrate Laravel with ChatGPT.
To get started, you’ll need to sign up for an API key from OpenAI, the company behind ChatGPT. You can do that by following these steps:
- Go to https://beta.openai.com/signup/
- Enter your email address and click “Get Started”
- Follow the prompts to create an account and sign in
- Once you’re signed in, click on the “API Keys” tab in the left sidebar
- Click the “Generate New Key” button to create a new API key
- Copy the key to your clipboard
Next, you’ll need to install the OpenAI API package in your Laravel project. You can do that using Composer by running the following command in your terminal:
composer require openai/api
Once the package is installed, you can use it to interact with ChatGPT. Here’s some sample code that you can use to get started:
use OpenAI\Api\Client;
use OpenAI\Exception\ApiException;
// Set up the API client
$client = new Client('your_api_key');
// Define the parameters for the chat request
$params = [
'model' => 'text-davinci-002',
'prompt' => 'Hello, how are you doing today?',
'temperature' => 0.5,
'max_tokens' => 50,
];
// Send the chat request to ChatGPT
try {
$response = $client->completions->create($params);
$message = $response['choices'][0]['text'];
} catch (ApiException $e) {
// Handle any errors that occur
$message = 'An error occurred: ' . $e->getMessage();
}
// Output the response from ChatGPT
echo $message;
This code sends a prompt to ChatGPT using the text-davinci-002
model and returns the response. You can modify the prompt and other parameters to suit your needs.
I hope this helps! Let me know if you have any questions.