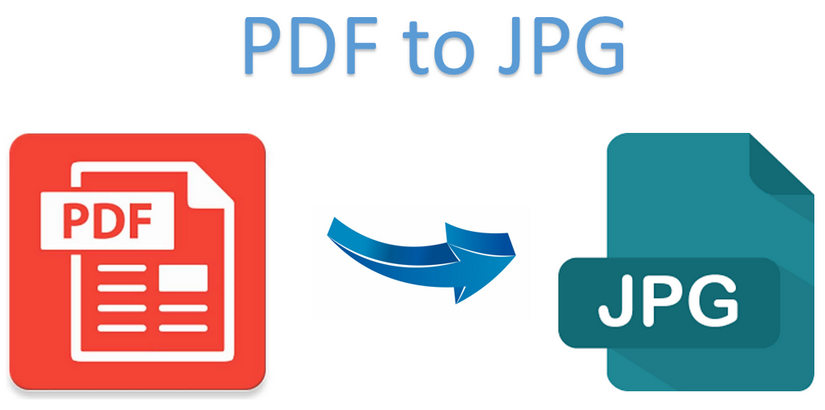
Many tools are available on the internet for converting a PDF to an image. Here we going to write code to convert PDF to IMAGE in python3.
Package required: – pdf2image
pip3 install pdf2image
Installing poppler : –
For Ububtu : – sudo apt-get install poppler-utils
For Archlinux : – sudo pacman -S poppler
For MacOS : – brew install poppler
For Windows : –
- Download the latest package from http://blog.alivate.com.au/poppler-windows/
- Extract the package
- Move the extracted directory to the desired place on your system
- Add the bin/ directory to your PATH
- Test that all went well by opening cmd and making sure that you can call pdftoppm -h
For Windows : –
from pdf2image import convert_from_path
import os
import sys
def convert_image(input_path, output_path):
new_output_path = output_path +'/output'
if not os.path.exists(new_output_path):
os.makedirs(new_output_path)
pages = convert_from_path(input_path , 100, poppler_path = r"E:\\poppler\\bin")
count = 0
for page in pages:
count=count+1
page.save(new_output_path +'/'+ str(count) +'.jpg', 'JPEG')
input_path = sys.argv[1]
output_path = sys.argv[2]
convert_image(input_path, output_path)
For Others : –
from pdf2image import convert_from_path
import os
import sys
def convert_image(input_path, output_path):
new_output_path = output_path +'/output'
if not os.path.exists(new_output_path):
os.makedirs(new_output_path)
pages = convert_from_path(input_path , 100)
count = 0
for page in pages:
count=count+1
page.save(new_output_path +'/'+ str(count) +'.jpg', 'JPEG')
input_path = sys.argv[1]
output_path = sys.argv[2]
convert_image(input_path, output_path)
Exceptional and also extremely interesting blog site.
Will certainly check out much more from now on.
excellent and amazing blog site. I actually wish to thanks, for giving us better information.
Excellent post. Articles that have purposeful and also informative content are more satisfying.