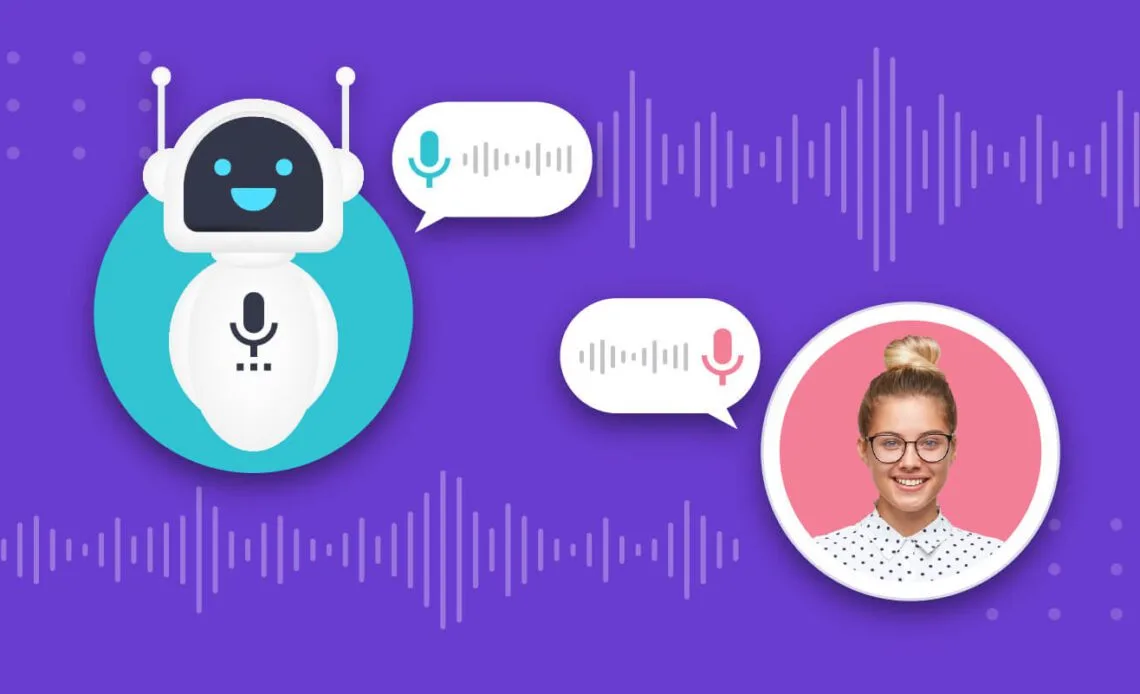
Introduction
Voicebots are becoming increasingly popular for automating customer service, providing information, and more. In this tutorial, we will build a voicebot using ChatGPT, Node.js, and Twilio. The voicebot will take user inputs via a phone call and respond using the GPT-4 language model from OpenAI.
Prerequisites
Before we start, make sure you have the following:
- Node.js installed on your machine.
- Twilio Account with a purchased phone number.
- OpenAI API Key for accessing ChatGPT.
Step 1: Setting Up Your Node.js Project
First, create a new Node.js project.
mkdir voicebot
cd voicebot
npm init -y
Install the necessary dependencies:
npm install express twilio axios body-parser
Step 2: Configuring Twilio
Log in to your Twilio account and navigate to the Phone Numbers section. Purchase a phone number if you haven’t already. Then, set up the webhook for incoming calls:
- Go to your phone number settings.
- Under the Voice & Fax section, set the A CALL COMES IN option to
Webhook
. - Enter your server URL (we’ll set this up in the next steps) and append
/voice
to it. For example,https://your-domain.com/voice
.
Step 3: Building the Express Server
Create a file named index.js
and set up a basic Express server:
const express = require('express');
const bodyParser = require('body-parser');
const axios = require('axios');
const { twiml: { VoiceResponse } } = require('twilio');
const app = express();
app.use(bodyParser.urlencoded({ extended: false }));
const port = process.env.PORT || 3000;
app.post('/voice', async (req, res) => {
const response = new VoiceResponse();
const gather = response.gather({
input: 'speech',
timeout: 5,
action: '/gather'
});
gather.say('Hello! How can I assist you today?');
res.type('text/xml');
res.send(response.toString());
});
app.post('/gather', async (req, res) => {
const speechResult = req.body.SpeechResult;
// Send the user's speech input to ChatGPT
const gptResponse = await axios.post('https://api.openai.com/v1/engines/davinci-codex/completions', {
prompt: `User: ${speechResult}\nAI:`,
max_tokens: 100,
temperature: 0.7,
n: 1,
stop: ["\n"]
}, {
headers: {
'Authorization': `Bearer YOUR_OPENAI_API_KEY`
}
});
const responseText = gptResponse.data.choices[0].text.trim();
const response = new VoiceResponse();
response.say(responseText);
const gather = response.gather({
input: 'speech',
timeout: 5,
action: '/gather'
});
gather.say('Is there anything else you would like to ask?');
res.type('text/xml');
res.send(response.toString());
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Replace YOUR_OPENAI_API_KEY
with your actual OpenAI API key.
Step 4: Deploying Your Server
You can deploy your server on platforms like Heroku, Vercel, or any other Node.js hosting service. Make sure your server is accessible over the internet and update the Twilio webhook URL accordingly.
Step 5: Testing Your Voicebot
Call your Twilio phone number and interact with your voicebot. You should be able to speak to it, and it will respond using the ChatGPT model.
Conclusion
Congratulations! You’ve successfully built a voicebot using ChatGPT, Node.js, and Twilio. This bot can be further enhanced by adding more complex conversational logic, integrating with databases, or connecting to other APIs. Voicebots have many applications, from customer service automation to personal assistants.