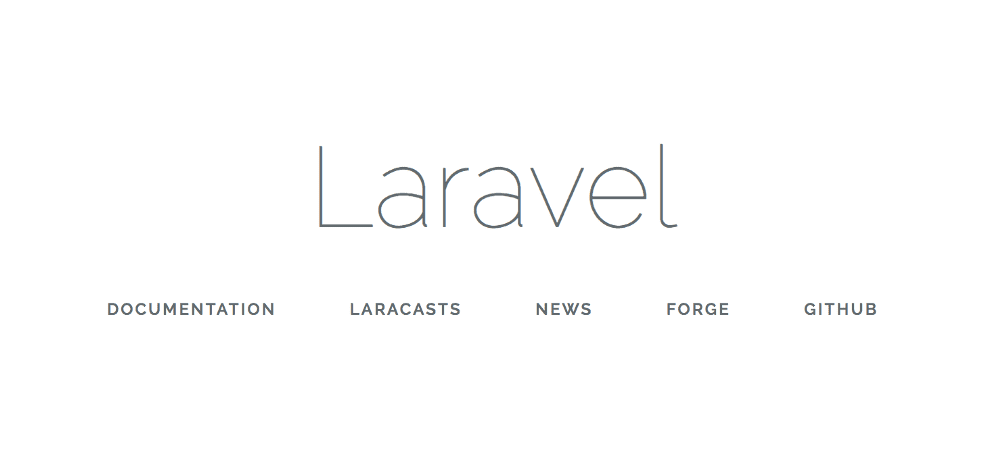
Advanced Container for Laravel is a package that provides syntax sugars for Laravel container calls, bindings, and more.
Here’s an example of binding services, singletons, and scoped interfaces with this package:
// Basic binding
bind(ServiceInterface::class)->to(Service::class);
// Singleton
bind(ServiceInterface::class)->singleton(Service::class);
// Scoped instance
bind(ServiceInterface::class)->scoped(Service::class);
With this package, you can do method binding using the package’s syntax sugar to do things like perform calls to your service through the container and override method behavior:
// Basic call to a service through the container
call(Service::class)->yourMethod(100)
// Override the method behavior
bind(Service::class)->method()->yourMethod(function ($service, $app, $params) {
return $service->yourMethod($params['count']) + 1;
});
// Alternative syntax to bind method behavior
bind(Service::class)->method('yourMethod', function ($service, $app, $params) {
return $service->yourMethod($params['count']) + 1;
});
Using this package, you can easily mock methods in your tests as well:
bind(ServiceInterface::class)->method(
'externalApiRequestReturnsFalse',
fn () => false
);
$service = call(ServiceInterface::class);
$call = $service->externalApiRequestReturnsFalse();
$this->assertFalse($call);
This package also supports method forwarding—check the README for details. You can learn more about this package, get full installation instructions, and view the source code on GitHub.