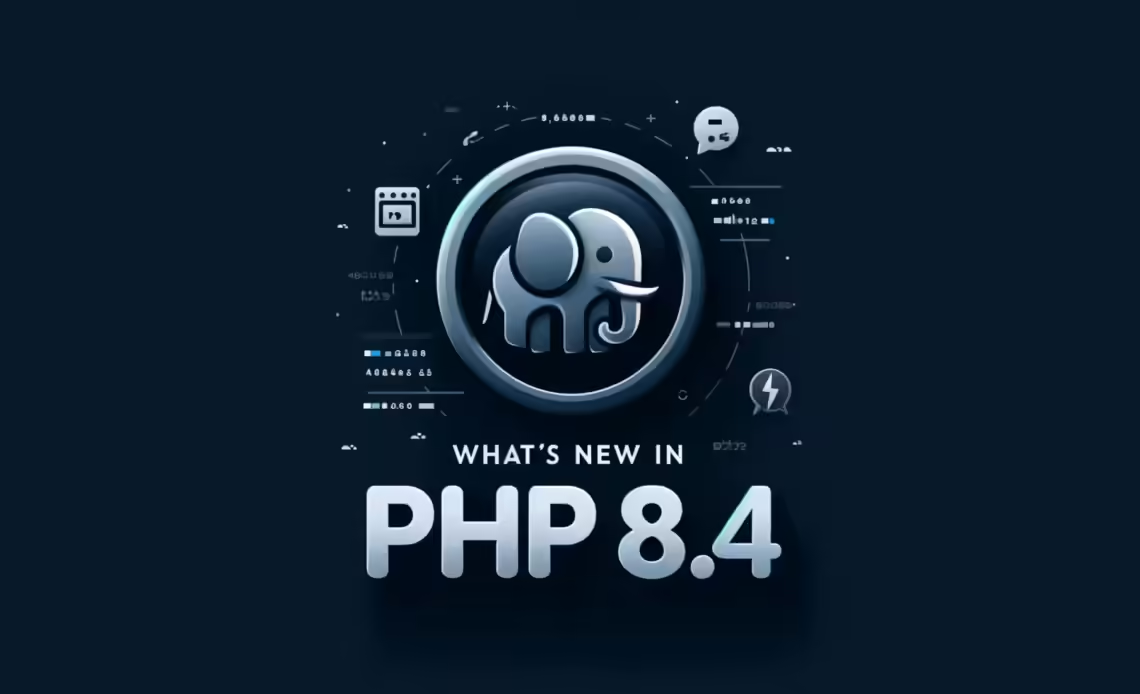
PHP 8.4 is set to be released on November 21, 2024, and it brings a host of exciting new features and enhancements that promise to make developers’ lives easier. From property hooks to simplified class instantiation, PHP 8.4 is packed with improvements that continue to push the language forward. In this post, we’ll explore some of the most anticipated features of PHP 8.4 and how they can benefit your development workflow.
When is PHP 8.4 Scheduled to be Released?
PHP 8.4 is scheduled for release on November 21, 2024. Before the official release, it will go through six months of pre-release phases, including Alphas, Betas, and Release Candidates. This structured rollout ensures that the final version is stable and polished, giving developers ample time to test and adapt to the new features.
PHP Property Hooks
One of the standout features in PHP 8.4 is property hooks. Inspired by languages like Kotlin, C#, and Swift, property hooks allow for more streamlined and efficient management of property access and updates. Here’s an example of how property hooks work:
class User implements Named
{
private bool $isModified = false;
public function __construct(
private string $first,
private string $last
) {}
public string $fullName {
// Override the "read" action with arbitrary logic.
get => $this->first . " " . $this->last;
// Override the "write" action with arbitrary logic.
set {
[$this->first, $this->last] = explode(' ', $value, 2);
$this->isModified = true;
}
}
}
With property hooks, you can remove the boilerplate code associated with property getters and setters, making your code cleaner and more concise. The ability to define access and update logic directly within the property is a significant enhancement that simplifies object-oriented programming in PHP.
For a deeper dive into property hooks, check out our detailed post: Property Hooks in PHP 8.4.
New new MyClass()->method()
Without Parentheses
In previous PHP versions, accessing class members immediately after instantiation required wrapping the new MyClass()
call in parentheses. This often led to parse errors if parentheses were omitted. PHP 8.4 addresses this issue by allowing direct member access without the need for extra parentheses:
// Wrapping parentheses are required to access class members in <= PHP 8.3
$request = (new Request())->withMethod('GET')->withUri('/hello-world');
// PHP Parse error (<= PHP 8.3): syntax error, unexpected token "->"
$request = new Request()->withMethod('GET')->withUri('/hello-world');
// In PHP 8.4, the above will be valid
$request = new Request()->withMethod('GET')->withUri('/hello-world');
This update eliminates a common source of frustration, making code more intuitive and aligning PHP syntax with other C-based languages like Java, C#, and TypeScript, which do not require surrounding parentheses for member access after instantiation.
For more information on this syntax change, read our detailed post: Class Instantiation Without Extra Parenthesis in PHP 8.4.
Conclusion
PHP 8.4 is poised to bring substantial improvements that enhance both the developer experience and code efficiency. Property hooks and the simplified class instantiation syntax are just two of the many features that will make PHP development more enjoyable and productive. As we approach the release date, keep an eye out for more updates and start planning how you can leverage these new capabilities in your projects.
Stay tuned for more in-depth articles and tutorials on PHP 8.4 features. Happy coding!