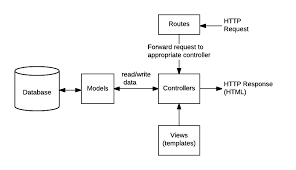
The Laravel team released 8.80 with the ability to define a route group controller, render a string with the Blade compiler, PHPRedis serialization and compression config support, and the latest changes in the v8.x branch.
Specify a Route Group Controller
Luke Downing contributed the ability to define a controller for a route group, meaning you don’t have to repeat which controller a route uses if the group uses the same controller:
Route::controller(PlacementController::class)
->prefix('placements')
->as('placements.')
->group(function () {
Route::get('', 'index')->name('index');
Route::get('/bills', 'bills')->name('bills');
Route::get('/bills/{bill}/invoice/pdf', 'invoice')->name('pdf.invoice');
});
Render a String With Blade
Jason Beggs contributed a Blade::render()
method that uses the Blade compiler to convert a string of Blade templating into a rendered string:
// Returns 'Hello, Satish'
Blade::render('Hello, {{ $name }}', ['name' => 'Satish']);
// Returns 'Foo '
Blade::render('@if($foo) Foo @else Bar @endif', ['foo' => true]);
// It even supports components :)
// Returns 'Hello, Sharma'
Blade::render('<x-test name="Sharma" />');
PHPRedis Serialization and Compression Config Support
Petr Levtonov contributed the ability to configure PHPRedis serialization and compression options instead of needing to overwrite the service provider or define a custom driver.
The PR introduced the following serialization options:
- NONE
- PHP
- JSON
- IGBINARY
- MSGPACK
And the following compressor options:
- NONE
- LZF
- ZSTD
- LZ4
These options are now documented in the Redis – Laravel documentation.